Learning Objectives
ENGR 132
Learning objectives outline the learning goals in this course. Use them to understand how your work will be assessed and to ensure your work meets the course expectations.
MATLAB Learning Objectives
MAT01: Programming Standards
Develop MATLAB code that follows professional programming standards.
Review the Programming Standards page for more details.
Evidences for proficiency
- Include a program header with assignment information, author and contributor information, program description, and help lines for functions (i.e., function call, input definitions with units, and output definitions with units). The program description should be clear (i.e., can be understood without confusion), concise (i.e., not redundant or superfluous), and complete (i.e., fully describe the program’s purpose).
- Use only variables that are initially assigned within the program or entered into the program via a function input. Note: it is considered improper variable assignment if a data file is imported into the Workspace outside the program.
- Place code in appropriate sections (i.e., initialization, calculations, plots and formatted text, etc.).
- Comment blocks of related code with clear and concise descriptions (see 1 above). Comment variable initializations with descriptions and units.
- Appropriately indent blocks of code within non-sequential structures; individual commands fit within single lines or are appropriately split into multiple lines; include spaces between variables and operations in calculations to enhance readability of code.
- Variable names should be valid and descriptive and should not overwrite built-in MATLAB functions or values.
- Suppress calculated values and commands with the semicolon operator. Note: plotting and printing commands do not need to be suppressed.
- Only perform hardcoding for storage of constant variables, index values, or for constants within calculations. Note: it is considered hardcoding to manually input data that should be imported from a data file using a MATLAB function.
Grading process
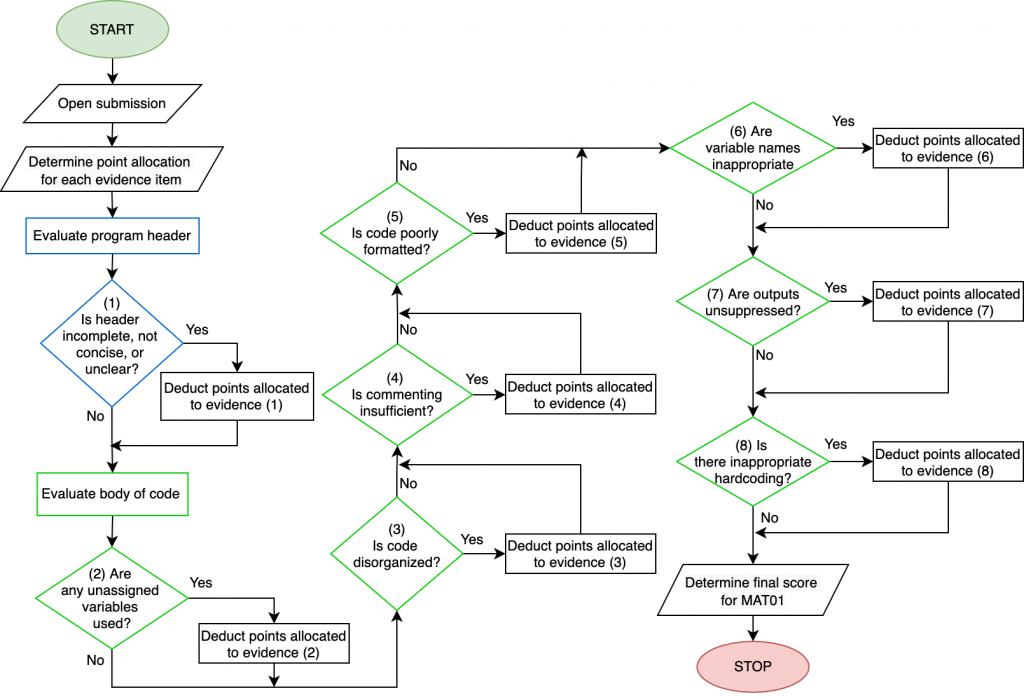
General note: For items (3)–(8), point deduction is based on general trends. If there are four or more opportunities to demonstrate a skill within a program, it is okay for there to be a mistake (e.g., one missing or poor comment out of many). However, if there are three or fewer instances (e.g., only one value that could be hardcoded), then failing to demonstrate the skill should lead to a point deduction.
MAT02: Data Storage
Store and access data within MATLAB
Evidences for proficiency
- Assign the results from any calculations or built-in function into variables. Assignment must occur from right to left.
- Do not overwrite original data sets by storing new values unless explicitly instructed; do not store the results of operations that do not produce meaningful outputs.
- Use proper indexing and syntax to copy or access the appropriate values from the data or built-in function. This may refer to accessing individual values or groups of values from vectors or matrices, or copying data from an imported data set.
- Create properly sized MATLAB arrays, including scalars, vectors, and matrices.
Grading process
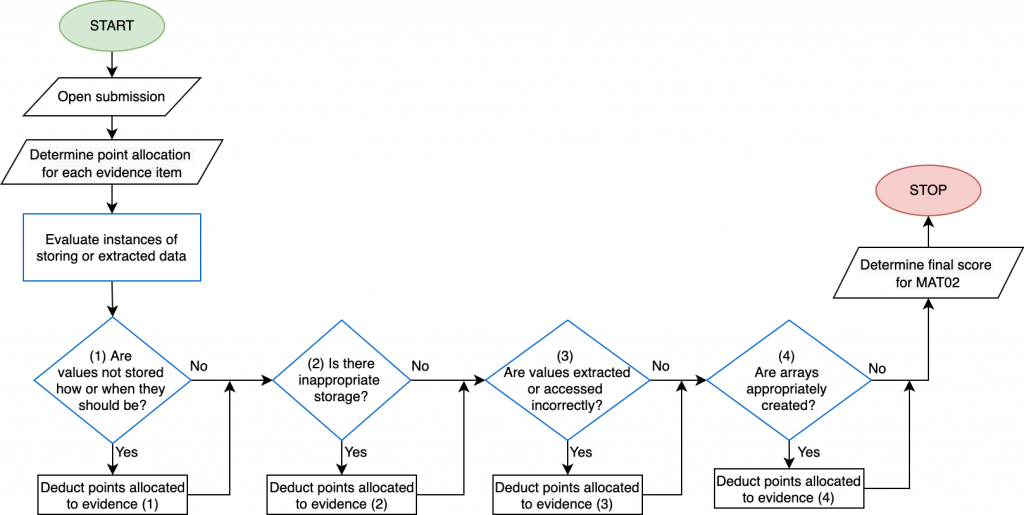
General note: When used with MAT08, isolated incidents of errors should not result in a loss of points. Points are deducted when there is an indication that the problem is persistent (at least 25% of the time), suggesting the work has not attained the necessary level of mastery for this learning objective (result accuracy is accounted for with MAT08).
MAT03: Calculations
Perform mathematical operations and calculations
Evidences for proficiency
- Use built-in MATLAB functions (e.g., statistical, trigonometric, algebraic, i/o, matrix transpositions, matrix concatenation,
disp
,fprintf
, plotting commands) when appropriate and with proper syntax. - Calculations must use the correct operations (e.g., addition, subtraction, multiplication, division) and values required by the problem. That is, if
slope*x_var + intercept
is needed,intercept*x_var + slope
orslope*x_var - intercept
would be incorrect.- This includes using correct values within a built-in function.
- Perform the steps in a calculation using precedence and parentheses to ensure the correct order of operations.
- This is for arithmetic calculations; order of operations/precedence for logical and relationship operations is handled in (5).
- This differs from the previous item, where order can be appropriate, but missing parentheses may result in incorrect results (e.g.,
3*2 + 5
versus3*(2 + 5)
).
- Use period characters to achieve element-by-element operations when, and only when, necessary in performing operations with vectors or matrices.
- Write logical and relational statements that use correct operators (e.g.,
&
,|
,&&
,||
,>
,==
, etc.) and syntax to produce accurate outcomes when comparing values or arrays.- It is usually okay if a logical/relational statement differs from the solution if it is ultimately logically equivalent (i.e., produces the same results).
Grading process
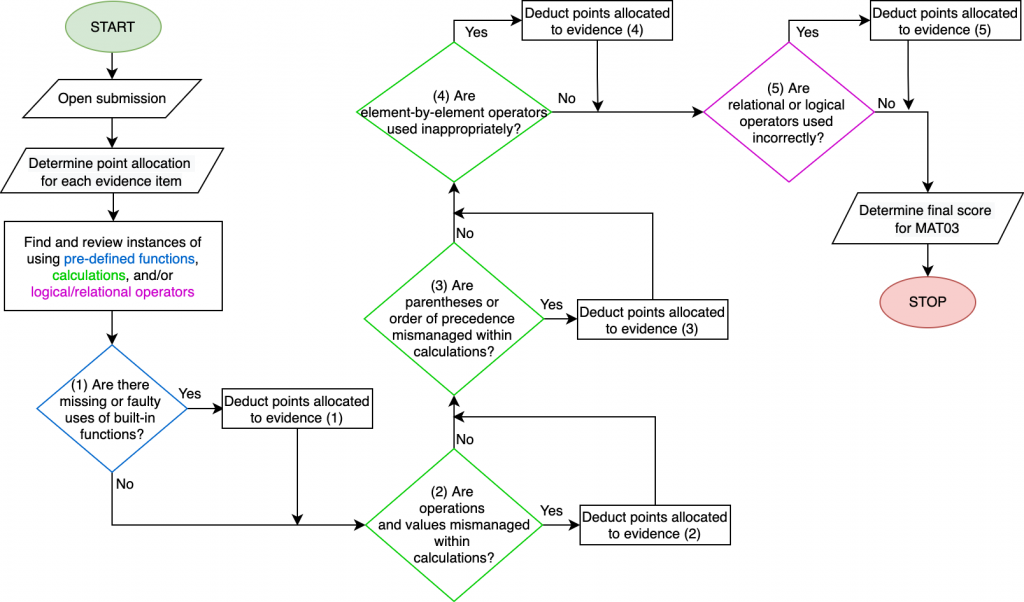
General note: When graded with MAT08, performance may be judged based on overall trends. If error occurs in 25% or fewer of instances, it may be disregarded.
MAT05: Scripts and Functions
Create and use MATLAB scripts and user-defined functions
Evidences for proficiency
- Begin all user-defined functions with a function definition line that starts with the keyword
function
and places inputs, outputs, and function name in proper order. - Use input and output arguments that are appropriate for the problem.
- If an external value must be used by the function, it must be included in the definition. If a variable is in the input list, it must be used within the function.
- If an output value is expected, it must be in the function definition and it must be created within the function.
- Recognize that information displayed to the Command Window by a function is not an output argument.
- Recognize that variables defined through the use of the
input
command are not equivalent to a user-defined function’s input arguments.
- Appropriately pass information through programs. Place functions in an appropriate order.
- Understand that a function has its own local workspace and the only way to pass information between workspaces is through input and output arguments.
- If functions are provided, then correctly track information through them.
- Create user-defined functions and use them to remove sequences of code that would otherwise be frequently repeated within a script or function.
- Call any script or function with the correct name, using expected number and order of inputs and storing into variables any expected outputs, when relevant.
- Do not use the keyword
function
in a function call. - Define input arguments prior to the function call, when relevant.
Grading process
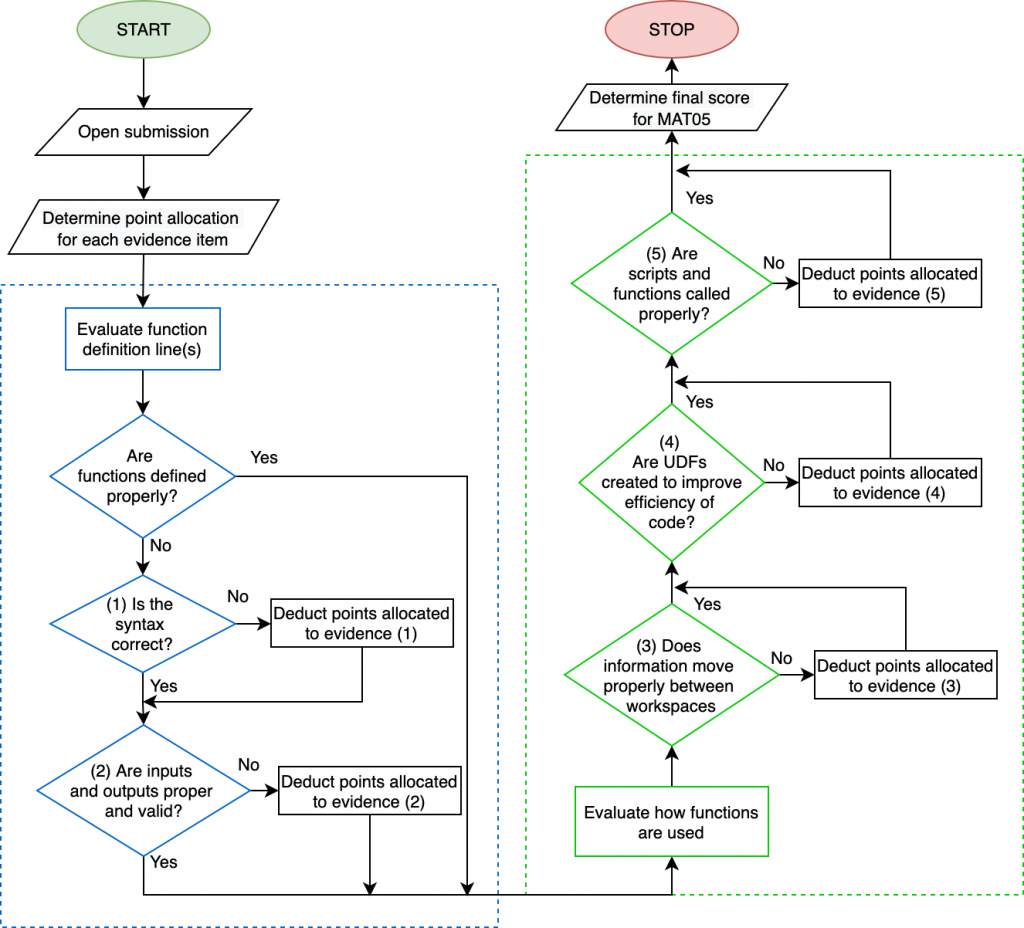
General note: When graded with MAT08, these evidences may be graded for general trends. Points should not be deducted for random, isolated errors unless that represents the only time the skill is demonstrated. This intends to identify if evidence exists that student is not yet proficient.
MAT06: Code Structures
Understand and write sequential and non-sequential code structures in MATLAB to achieve a programming objective
Evidences for proficiency
- Perform operations in the appropriate order to achieve the goal of the block of sequential code and to produce the intended results.
- Make an appropriate choice between a selection or a repetition structure given the context of the problem. An effective selection structure will establish the number of paths required by the problem. If a specific repetition structure is specified in the problem, that should be used; otherwise, if a
while
is used when there is a known number of iterations, the overall structure must be designed to iterate the correct number of times. - Enter the structure using a condition or control statement that meet the demands of the context of the problem, such that a true statement enters the structure. Variables used in condition statements for selection and indefinite repetition structures (i.e.,
while
) must be defined prior to structure. Definite looping structures use or define a vector that iterates an appropriate number of times for the context. - Perform operations within the non-sequential structure by using previously defined variables and by placing operations appropriately within the structure (i.e., only contain operations that are specific to the condition or repetition structure—structure does not contain operations that could exist outside of the structure; however, all operations necessary for the condition or loop are included). This includes nesting of non-sequential structures.
- There is an
end
corresponding to each non-sequential structure. Indefinite looping structure conditions will become false.
Grading process
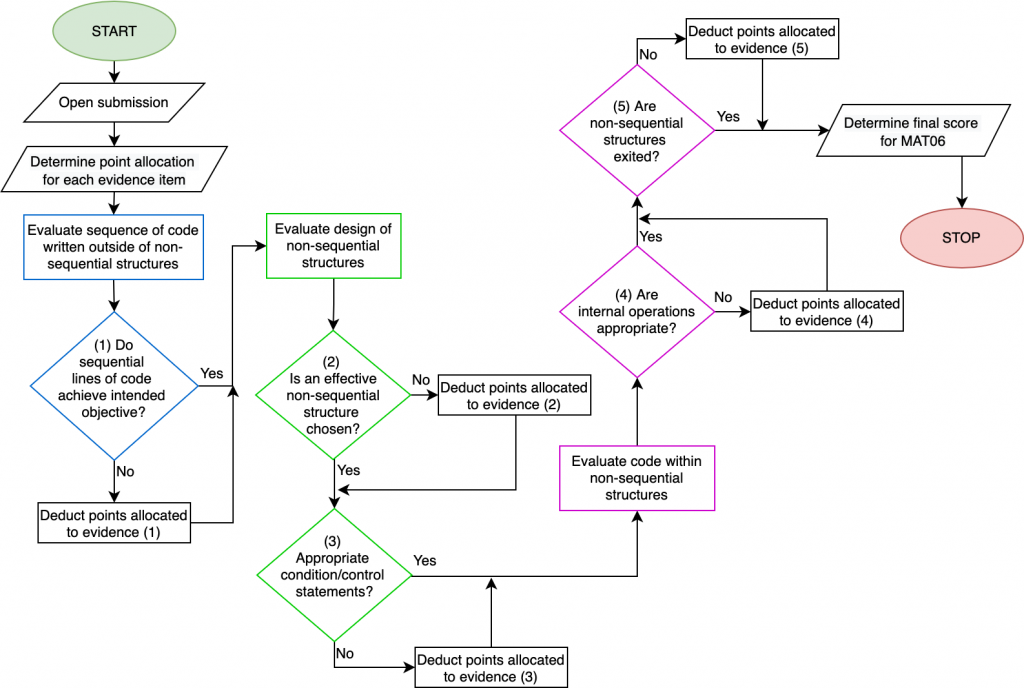
General note: When used with MAT08, these evidences are examined for general trends. Points should not be deducted for random, isolated errors unless that represents the only time the skill is demonstrated. This intends to identify if evidence exists that student is not yet proficient.
MAT07: Translate Program Descriptions
Translate language descriptions and programming flowcharts to develop MATLAB code
Evidences for proficiency
- Parallelograms correspond to input/output operations; rectangles correspond to calculations or non-output operations; ovals correspond to the beginning or end of the code.
- Diamonds with one input and two outputs correspond to selection structures and differentiate between an
if
diamond andelseif
diamond(s); diamonds with two input arrows and two output arrows correspond to repetition structures, with definite structures containing three internal components. - Translations of non-sequential structures have proper conditions or control statements that allow entry to the proper path (selection structures) or result in the correct number of iterations (repetition structures).
- Necessary commands, such as
else
orend
, are properly placed within the translation. - Maintain indicated order of operations from description to flowchart or flowchart to code. That is, operations indicated as being within a non-sequential structure appear within the non-sequential structure (i.e., operations on the ‘yes’ path) and the order in which operations are performed is not changed.
- If code is being written from a flowchart, each element within the flowchart should be included in the code. If a flowchart is being created from a description, each aspect of what the program should accomplish is accounted for by the flowchart. The translation should not include additional elements that are not part of the flowchart or description.
Grading process
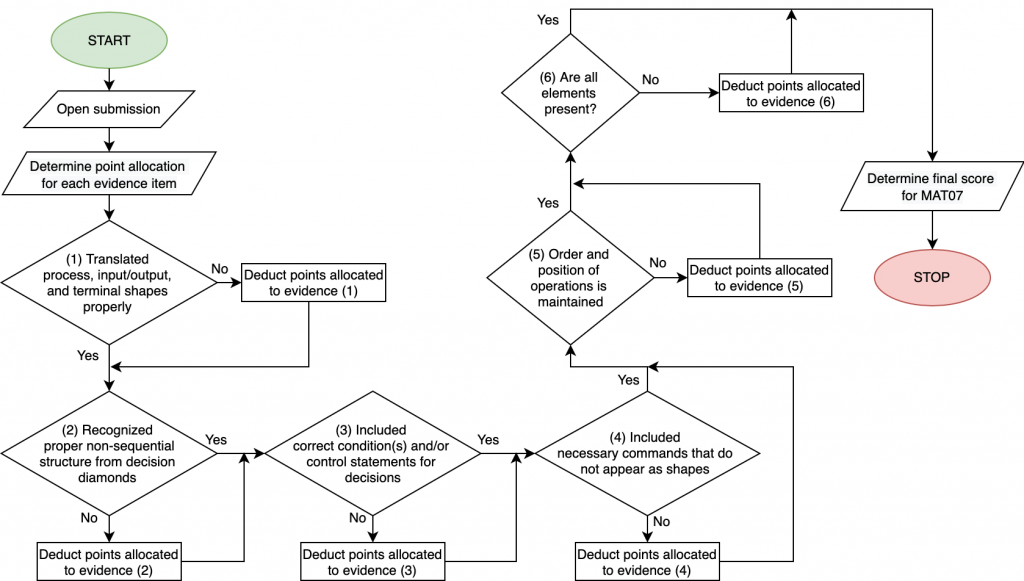
General note: When used with MAT08, these evidences are examined for general trends. Errors should lead to point deductions if they constitute 25% or more of the instances in which the skill should be demonstrated. This intends to identify if evidence exists that student is not yet proficient.
MAT08: Debugging
Produce code that achieves its objective without generating dynamic or semantic errors
Evidences for proficiency
- The program must not have procedural issues. Procedural issues generally refer to completing the program using methods that differ from directions given in the problem (e.g., a for-loop is directed explicitly in the problem but a while-loop is used).
- For closed-ended problems, script/function should produce the same exact outputs as was specified in the solutions. For open-ended problems, results of script/function should be appropriate based on the parameters of the problem and should fall within the range of acceptable answers suggested in the solutions. Errors could be the result of a misinterpreted problem context (e.g., thinking the problem gave a diameter instead of a radius). This is where that error will lose points, not elsewhere in the grading.
- The program must run without any unplanned errors (e.g., without incorrect function names, missing parentheses, or missing ‘end’ statements).
Grading process
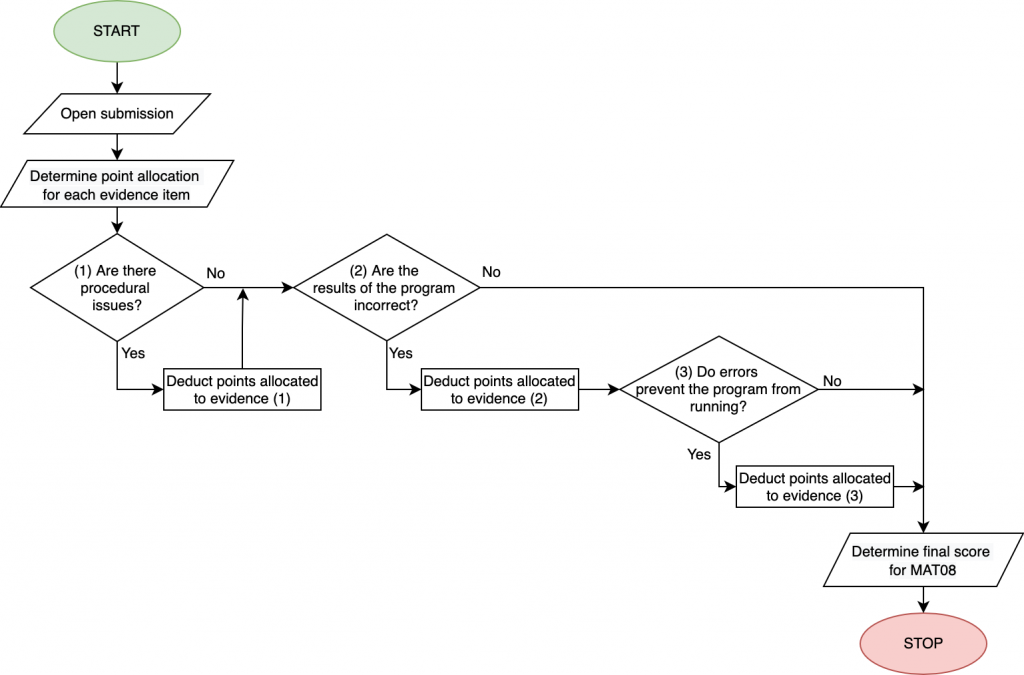
MAT09: Test and Track Code Structures
Test and track information through flowcharts and programs
Evidences for proficiency
- Include written descriptions of test cases or outcomes using comprehensible English and list input values in an appropriate format. Descriptions and values correspond to one another (i.e., temperature for “Freezing” should be below 0 C).
- For individual input values, state the correct outcomes. For tracking table, state correct value at each line. For a test case table, include all necessary test cases with a correct outcome for each. Necessary test cases are dictated by the context of the problem.
- Correctly identify which paths are taken through the code or flowchart. “Algorithm” can refer to code or flowchart. May refer to tracking any input value or test case.
- This does not relate to number of iterations through repetition structure—that is dealt with in evidence 4.
- Detail a full set of test cases or tracking results for the provided program or flowchart. May refer to tracking table or test table. For a tracking table through a repetition structure, this means identifying the correct number of iterations. For a test case table, it means having at least one test case for any given path. Disregard for individual test values.
Grading process
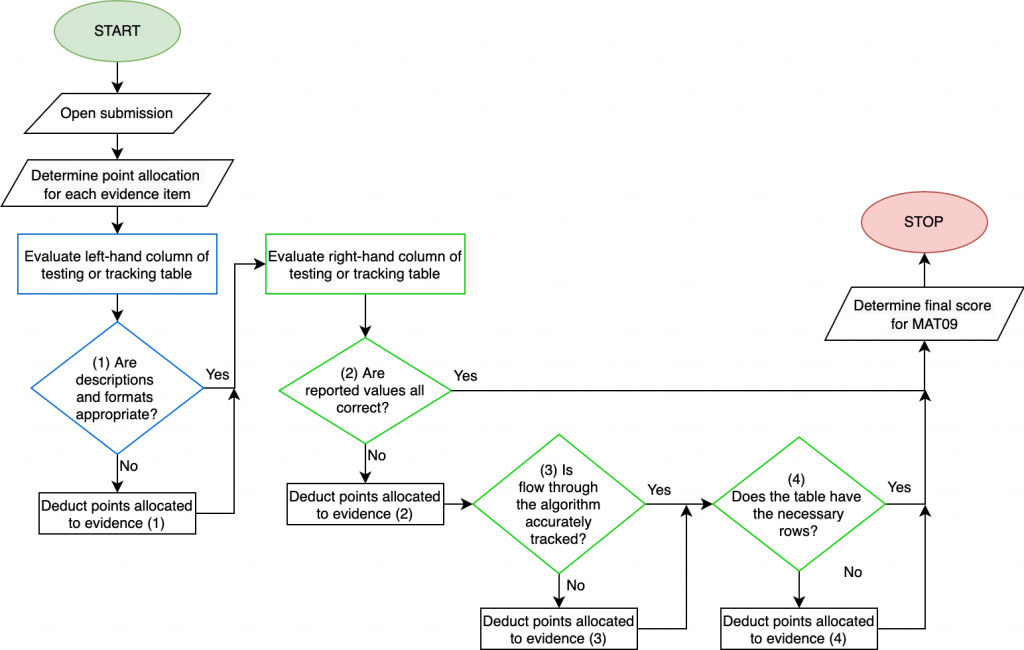
Modeling Learning Objectives
MOD01: Mathematical Modeling
Select and determine mathematical models for data
Evidences for proficiency
- The final model has proper function form and parameters.
- For linear models of untransformed (i.e., original) data, this means presenting a linear model in the form y = mx+b, where m and b are the slope and intercept, respectively.
- For non-linear models of untransformed data, this means presenting a final model with an appropriate form for the function type with proper parameters m and b:
- Exponential: y=b*10^mx
- Logarithmic: y=m*log(x)+b
- Power: y=b*x^m
- For linearized models of transformed (i.e., linearized) data, this means presenting the model in the form Y=MX+B, where coefficients M and B are the slope and intercept of the linearized data and X, Y are the appropriately linearized data.
- When done in MATLAB, this evidence may be graded in MAT08 instead of in MOD01
- Present a model that is the function type that best fits the original data (i.e., exponential, linear, logarithmic, or power).
- For non-linear data: Transform the original data into linearized data using appropriate log functions for the desired model type.
- Exponential data: linearize by taking the log of the y data only
- Linear data: do not transform the data
- Logarithmic data: linearize by taking the log of the x data only
- Power data: linearize by taking the log of the x data and the log of the y data
- For non-linear modeling: Properly determine the coefficients, M and B, for a linear model of the transformed (i.e., linearized) data. This could mean using built-in functions (i.e.,
polyfit
andpolyval
in MATLAB) or using the least-squares calculations. The calculations require the use of linearized data. - For non-linear modeling: Properly convert the linearized coefficients M and B into the function parameters m and b, and place properly in the general function form.
Grading process
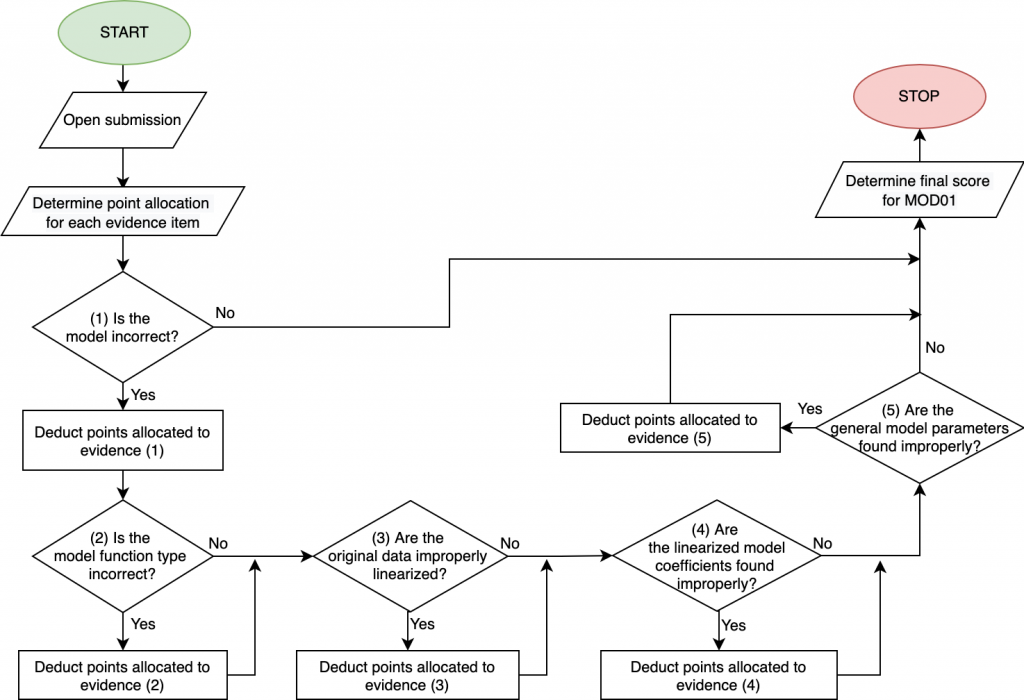
Engineering Professional Skills
EPS01: Professional Communication
Use professional written and oral communication
Evidences for proficiency
- Make arguments and claims that are logically consistent with the results that were found (e.g., even if accurate answer suggests an increasing trend, if student’s calculations show a decreasing trend, they should claim that the values are decreasing).
- Support arguments by using relevant and explicit reference to pertinent information, data, or results (e.g., reference slope when discussing change; properly reference coefficient of determination when justifying strength of model; explain model limitations with reference to data set range when making predictions)
- Present numerical values with an appropriate number of decimal places.
- Include appropriate descriptions, labels, or units with responses and results.
- Responses need to be in full, complete sentences using clear and concise language with a tone that is appropriate for a professional or academic engineering setting and should be proofread to be free of spelling and grammatical errors. Clear descriptions can be understood without confusion and concise descriptions are not redundant or superfluous. Professional text formatting is expected in written communication.
- When displaying text using coding commands, ensure the display is readable within the tool (i.e., use appropriate line breaks to keep text visible with no horizontal scrolling and to move the command prompt to a new line after the final text statement.
Grading process
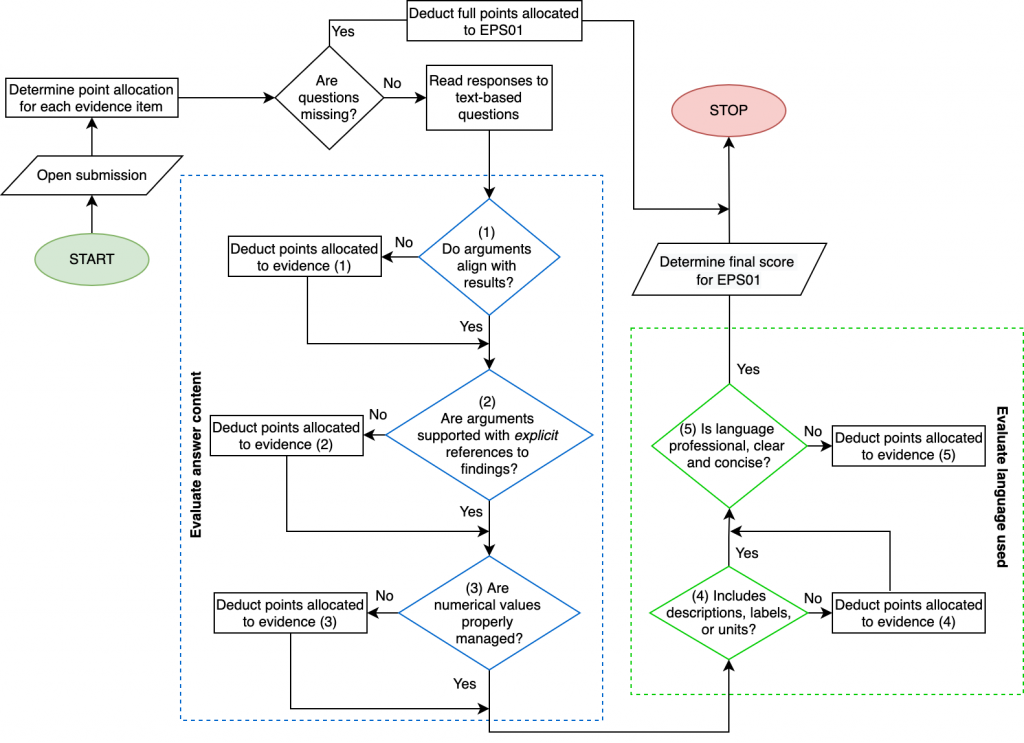
EPS02: Professional Plot Formatting
Create professional graphical representations of data and models
Evidences for proficiency
- Manage multiple plots as directed into separate figures, overlay plots, or subplots when presenting multiple plots. Plots should be organized following the exact directions in the assignment (i.e., correct figure numbers, order of plots within subplots).
- Include descriptive titles to fully and effectively communicate content. Concise titles are not redundant. Titles should differentiate subplots. Remember the title convention “y-data vs x-data”. Err on the side of accepting titles that are over-descriptive rather than under-descriptive.
- Use appropriate axis labels to communicate the correct variable being plotted and include accurate units. Pay close attention to units for data that may have been linearized.
- Display a legend with appropriate color and/or line style selections to clearly differentiate data sets.
- Place legends or model equations on the figure in a way that minimizes obstruction of data points.
- Select appropriate line and marker styles to convey modeled data or collected data (i.e., use data markers for collected data; use lines without markers for modeled data; use line-marker combinations when permitted to visualize trends in collected data).
- Orient axes with horizontal independent variable, scaled according to directions or proper selection (i.e., linear, logarithmic, etc.), and display gridlines.
Grading process
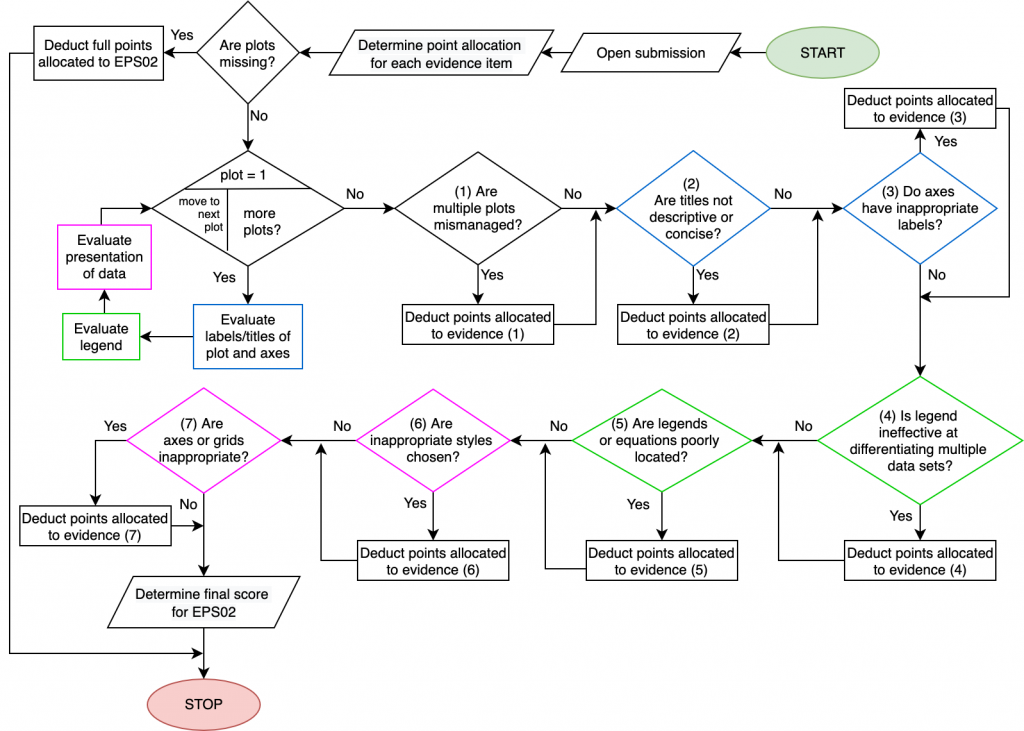
General note: General trends should be considered for (2)–(5) when several (i.e., more than 3) plots are included in the answer. However, (1), (6) and (7) may lose points for a single error among several plots.
PC05: Meet Assignment Expectations
Fully address all parts of assignment by following instructions and completing all work
Evidences for proficiency
- Attempt to solve the problem or demonstrate evidence of learning objectives.
- Submit deliverables on time.
- Submit all requested supporting files and information, such as data files, images, instruction text, or p-code files.
- Use appropriate file names or file types for deliverables.
- Add teammates as group members on group assignments (only for group assignments).
- Include name(s) and Career account login(s) where requested.
- Include Team ID number where requested.
- Follow any additional assignment-specific instructions.
Grading process
The grader will review a submission to ensure that expectations and requirements are met. Points are deducted if something is not completed as directed.