ECE 462 Group Assignment
Stage 1: Generate Tetris Pieces
Deadline: 11:59PM 2009/09/27
Everyone in the team must submit every stage of the assignment. If you do not submit and your teammates submit, you will receive only 80% of the score of the team. This requirement is used to prevent the situation when everyone in the team thinks someone else has submitted and actually nobody submitted.
Summary
Your team needs to build a program that can generate Tetris pieces, each with 4, 5, 6, or 7 squares.
Piece Generation
The original Tetris has 7 pieces, each with 4 squares, shown below

If mirror operation is allowed, there are only 5 unique pieces. The 'L' and 'Z' shapes and their counterparts are considered duplicates under mirror operation.

If we allow a piece to have 5 squares, there are more different pieces. The following figure shows some examples, 12 unique 5-square pieces. If two pieces are identical by mirror, only one is counted. Since Tetris does not allow mirror, there are more than 12 pieces, each with 5 squares. Instead of giving you the correct solution, the following figure shows a subset of what your program should generate.

We can further extend to pieces of 6 squares; there are 35 pieces, if rotations and mirrors are allowed.

There are 107 unique 7-square pieces, if rotations and mirrors are allowed. Some pieces are not shown here (notice the scrollbars).

The examples above are given under the assumption that mirror operation is allowed. However, you are to consider the case that mirror operation is not allowed in this assignment. This means, for examples, "|_" and "_|" are two different pieces since one cannot be obtained from another by rotation operation only. Your program should generate both of them.
Requirements
Your team needs to submit two programs. In your CVS repository (where you have CVSROOT), create a folder named "gpa1", then commit the two projects into two subdirectories of this directory. The directory structure looks something like this
projects // root of CVS repository
|-- CVSROOT // this is required by CVS
`-- gpa1
|-- piecegen // project files for Piece Generator
`-- prototype // project files for Tetris Interface
Click here if you do not know how to check in your project into a subdirectory inside your repository.
Piece Generator
- This program has a slider showing the number of squares in each piece, similar to the GUI shown above. When user change the slider value, the canvas shows the pieces each of which consists of the according number of squares.
- Besides the slider and the canvas, there is a menu item named "Export" allowing user to export the generated pieces to an ASCII file which they may specify through a FileChooser dialog box.
- Rules for generating pieces:
- Rotation is allowed but mirroring is not admissible (therefore the correct results are different from the examples given above). Two pieces are considered duplicates if one can become the other by rotation (90, 180, or 270 degrees) and should be avoided. This means that, for instance, "S" shape and "Z" shape are not equivalent. Under this assumption, there should be 7 (not 5) different pieces consisting of 4 squares. If one piece is equivalent to another by rotation or mirror, the two pieces are equivalent and only one is kept. For 4-square pieces, there is only one T.
-
In any generated piece, all squares are adjacent to (sharing an edge with) at least one of the other squares. This literally means that "broken" or "disconnected" pieces are not valid. In computer graphics we call this property 4-connected neighborhood.
-
Do not generate any piece with a hole. A hole is one or more square that is not occupied and is surrounded by squares in all four sides. The following is a 7-square piece with a hole.
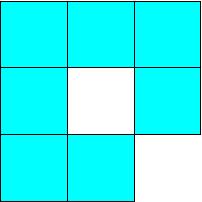
- You need to explain clearly how the pieces are generated in your documentation. You must have an algorithmic approach. Generating pieces by hand is not allowed. Do not intend to do so. There are nearly 200 distinct pieces. If you try to generate them by hand, you will very likely miss some and also have some duplicates. You must explain why your method can generate all possible pieces without any duplicates.
- Rules for exporting to file:
- Exportly only those pieces with the user-specified (by the slider) number of squares.
- Encoding: for an N-square piece, put the piece anywhere (need not touch the upper-left corner) in a N-by-N checkboard. Then, assign 1 to a check if it contains a square of the piece and 0 for empty checks. Finally, concatenate all N rows and form an N2-character string of '0's and '1's. An example of encoding a 5-square piece is illustrated below.
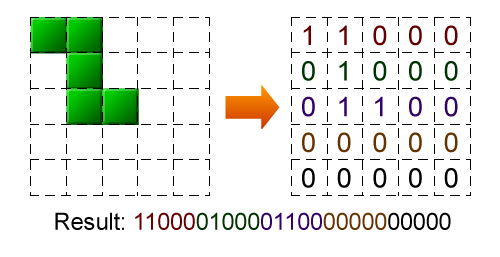
- Write the encoded string to the file in a single line. Each piece is represented by one line in the file.
- There is no specified order in which the piece should be exported.
Tetris Interface/Prototype
Enhance the Tetris (shown below) so that the pieces appear in a pre-determined order (not randomly). Your program should update only the next piece and the center panel. Ignore "Level", "Score", and "Removed Lines." The center panel has size 16 (width) and height 24 (height) squares. The pieces are chosen from 4-square pieces. After all 7 pieces have appeared, 5-square pieces appear. Then, 6-square pieces and 7-square pieces. In this stage, only one piece appears in the center panel. The piece moves down one square every 0.5 second. When the piece cannot move down any more (i.e. it touches the bottom), the piece disappears and the next piece enters the panel.

Grading
The full score of this stage is 6 points, divided into the following items.
Program Functionality - Piece Generator (3pt)
- (0.5pt) Graphical user interface - The programs runs, showing a window (resizeable) with a slider, a canvas and a menu item 'Export'. When the slider is dragged, the content in the canvas is updated accordingly.
- (0.7pt) Exporting to file - When the Export menu item is clicked, display a FileChooser dialogbox. After user specify a file name, the generated pieces are properly exported to the specified file in correct format.
- (0.8pt) Rendering pieces - The generated pieces are rendered in the canvas area with user-friendly look and feel, which means at least the following requirements are fulfilled:
- Pieces are correctly drawn (above all), preferably in an aesthetical way.
- If user stretch/shrink the window, the canvas size should change to fit the window.
- The size of each piece should be adaptive according to the number of pieces generated -- if there are a large number of pieces generated, make the pieces smaller; otherwise make them bigger for comfortable view.
- Scroll bars are shown whenever necessary. Consider the situation when user resizes the window.
- (1pt) Correctness of piece generating algorithm - 6-square and 7-square pieces are checked. 0.1 point is taken for each missing piece, incorrect piece or duplicate piece. You will not get a negative score for this category. This item is graded using the exported ASCII file, so naturally you will not receive any point if the export functionality does not work properly (or not implemented).
Program Functionality - Tetris Interface/Prototype (1.8pt)
- (0.6pt) The programs runs with a window containing at least a field named "Next Piece" and a playground (size: 16 squares x 24 squares). The field displays the piece that is about to enter the playground.
- (0.6pt) The piece falls one line every 0.5 second and vanishes when it touches the ground.
- (0.6pt) Pieces emerge in this order: enumerate all 4-square pieces with no duplicates (defined in [Requirements] for piece generator), then 5-square pieces followed by 6-square pieces. The piece emerges at the top of the playground. There is no requirement which orientation/angle the piece must be in.
- Mouse/keyboard interaction, scoring, start/pause functions are not required in this stage.
Program Readability (1pt)
Coding Style (0.5pt)
- (0.1pt) The code is formatted uniformly with proper indentation. Make your code look beautiful and professional. Use code formatter in your IDE (both NetBeans and Eclipse provide such function).
- (0.2pt) Classes, objects and members are named under coding conventions. There are several conventions for your choice to follow:
As there are many existing conventions for C++ coding, the principle of your coding style is to be consistent, understandable and easy to read.
- (0.2pt) There should be explanatory comments to help reader understand your code. Also, give a brief description about the class at the beginning of each class file.
Documentation (0.5pt)
- (0.2pt) Analyze your algorithm for piece generation. Give examples and/or illustrations to help elaborate your analysis.
- (0.2pt) Explain how your program works, what functions every class/object provides, etc.
- (0.1pt) Explain how your code is prepared for the next stage of development, i.e., what part of the code can be reused and how to extend your program to fulfill the requirements in the next stage.
Evaluation (0.2pt)
You will be asked to fill in an evaluation form to evaluate the performance of each team member (including yourself). This category will depend on the result of the evaluation from you and your partners. The aspects of the evaluation include
- providing meaningful contributions to the assignment (ideas, coding techniques, etc.)
- writing readable and maintainable code (coding style, comments and documentation style)
- attitude toward group affairs (organizing/attending group meetings, assigning duties, working cooperatively, etc.)
You will get 0.2 point if you finish the evaluation form.
Submission Procedure
- Follow the link or directions on the submission page of the assignment and fill in the peer evaluation form. The link will be available soon.
- Pack your code: put your source code in your CVS repository with correct directory structure and make a zip file of the repository using the following command:
zip -r gpa1.zip <cvsroot>\gpa1where <cvsroot> is the root directory of your CVS repository. Now two projects are packed into one zip file.
- Verify the zip file: open the zip file and verify that the names of the content files have trailing ",v" (comma v). This means that your submission is a package of CVS repository, not the unmanaged source files. If you do not submit CVS repository files, you will get zero for the assignment.
- Prepare your documentation file: acceptable formats of documentation files are PDF and README. If you use PDF, make sure your document can be rendered correctly in Linux environment. If you write ASCII documentation file, name it as README (anything else could result in grade penalty).
- Upload the zip file and the documentation file as two attachments on Blackboard. Make sure they are uploaded to the correct assignment slot.
- Download the files and double check they are not corrupted or mistakenly uploaded.
Submission of assignments is controlled by Blackboard system. If you fail to submit the correct files to the correct slot, you can always withdraw your submission until the final deadline. You cannot correct your submissions after the final due date, and your assignments are graded based on what is left on Blackboard system. No email submissions/corrections will be accepted.
Only CVS repositories will be accepted in this course. SVN repositories are not acceptable.
Should you have one panel for each piece?
Consider the following factors before deciding your answer.
- How to move a piece down or left or right?
- How to determine whether two pieces collide?
- How to eliminate a square because it belongs to a line that is completely filled?
Should each piece has it own panel?
If each piece has it own panel, how do you detect collsion?
Supplementary Materials
- The following link directs you to a detailed tutorial of CVS. If you do not know how to setup CVS using SSH, it could be very helpful (see "Local or Remote Access" section).
http://owen.sj.ca.us/~rk/howto/cvs.html (skip "CVS as a server" section)
- Do not rush to start coding. Take a look at some design principles of object-oriented programming (websites listed below) to have a general idea how you should organize the classes/objects. Discuss with your partners about your design and see if it meets these principles.
|