Structs
Goals
- Learn how use structures for data encapsulation
- Practice programming with dynamic memory (i.e., malloc)
- Practice debugging memory errors
- Learn how use structures for data encapsulation
- Practice putting a semicolon (";") after your struct statement :)
Overview
Scenario: Your team will soon choose a leader. You will be given a list of names and a separate list of each person's "favorite" person on the team. You must create software that can calculate the new leader.
Prepare
The following will help you get started:
-
Write some tiny programs using the following, to make sure you understand
each completely. Do not simply read existing examples. If you see
something online that you want to try, type it instead of
copy-pasting it in.
- struct declarations
- address of struct
- operators for accessing struct members: "." and "->"
- malloc() and free()
- operators for working with addresses: "*" and "&"
- Read the Code Quality Standards. Note the changes with regard to struct initialization, braces for blocks, and multiple statements on one line.
- As always… read this assignment description carefully.
There are no starter files.
Doing the assignment
Here is the overview of what you are creating.
- team.h
- struct Person type definition with at least two fields: name (string), favorite (the person's favorite, represented as the address of a struct Person object)
- struct Team type definition with at least two fields: people (array of Person objects), population (number of people on the team)
- forward declarations
- team.c
- create_team(int population, char* names(), int* favorites) function definition creates an instance of struct Team with population people. The favorite of the person named names[i] is favorites[i]. A person may be their own favorite.
- pick_leader(struct Team* team) function definition returns the most popular person, i.e., the person who is the favorite of the greatest number of people
- free_team(struct Team* team) function definition frees all heap memory referred to by the team (directly or indirectly, including the struct Person objects inside)
- teamtest.c
- main(…) function tests your functions in team.c; must be your own, and not the same as the own shown in the screenshot
Note: Type the declarations into your file manually. Do not copy-paste. (You learn more from typing code manually.)
When you are finished, it will look like this.
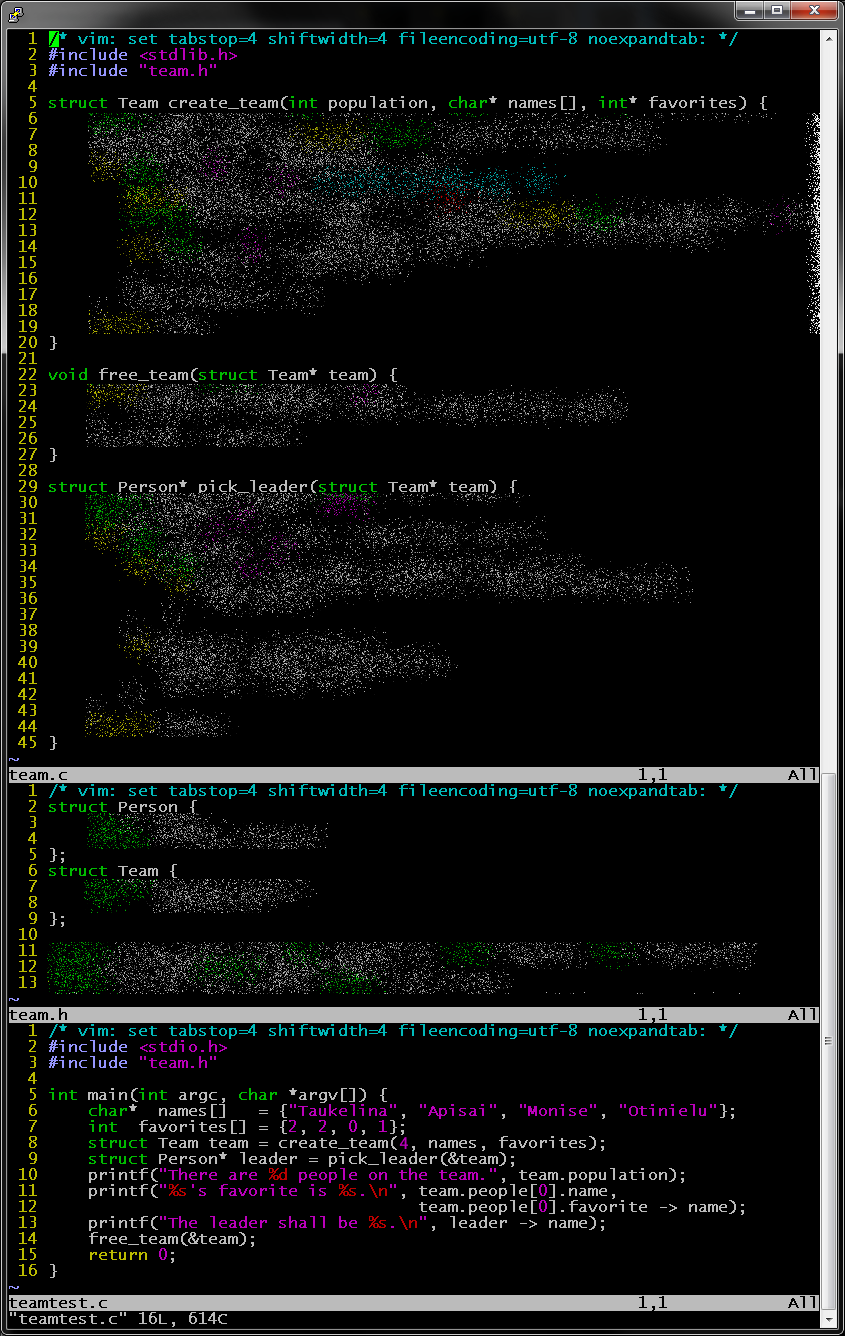
Requirements
- Your submission must contain the following files: team.c, team.h, and teamtest.c, with the functions and definitions as shown in the screenshot.
- Code must meet the code quality standards, including the new requirements regarding struct initialization, braces for if/for/while/do/switch blocks, and multiple statements.
- Do not use dynamic arrays (C99).
Do not use nested for loops.⇐ This requirement has been eliminated.- Your team.c may not include any header file except stdlib.h
- Your team.c may not call any function or refer to any external symbol except malloc and free.
- Your code may not make any assumptions about the size of any type.
- Code must compile without warnings or errors on ecegrid using the installed version of gcc installed there and the flags given in the provided .bashrc file (-std=c99 -pedantic -g -Wall -Wshadow).
- Code must run without crashing, for your test file and for any other valid test file.
- Your teamtest.c must be your own. The one shown is inadequate by itself. You must test the entire functionality of your code.
- If there is a tie in pick_leader, pick the one with the smallest index.
- A person may be their own favorite.
- Your code must work with the example code shown in the screenshot. You are welcome and encouraged to test with it, but give it a different name (e.g., example_teamtest.c) and do not copy-paste from that into your own teamtest.c.
- In create_team, you may assume that names and favorites are both valid arrays of size population.
How much work is this?
This assignment is difficult. It won't require a lot of code, but expect to spend some time debugging and understanding the concepts.
Updates
10/2: Added topics to practice, more description of files to be turned in, requirements 12-14; added reminder to requirement #2 about new code standards; fixed typo in screenshot (create_team(4, …))
10/3: Correction: favorite field of struct Person should be the address of another struct Person object
10/5: Eliminated requirement #4 ("Do not use for loops").
10/6: Clarified role of free_team(…).