ECE 462 Individual Programming Assignment
Breakout Game
Breakout Game
This game has many variations and some are available on line without charge, for example
 
You will learn how to create a computer game, including how to
- create a graphical user interface.
- handle user inputs.
- perform collision detection.
- save and retrieve game status using files.
Since this is the first C++ or Java program for many of you, you must write the program "from scratch." Do not download any open-source program and modify it for this assignment. This specification is given "as it is.". If anything is incomplete, inconsistent, or incorrect, please explain your interpretation or improvement of the specification in README. One of the purposes of this assignment is to provide an opportunity to learn how to handle an imperfect specification. You can discuss how to improve the specification by posting in Blackboard discussion.
Game Components
The game includes the following components. To simplify collision detection, everything is a circle.
- Playground: A white circle shows the playground. The radius of the circle is 300 pixels. The background color is black.

- Paddle: There is one semicircular paddle in the game moving horizontally. The paddle's radius is 30 pixels. In this figure, the blue semicircle is the paddle.
- Ball: It bounces after hitting the paddle, a boundary of the playground, or a brick. The bounce (also called reflection) follows the law of reflection: the angle of incidence equals the angle of reflection. If the ball falls below the paddle, the ball is lost and the game is over. In this figure, the red at the northwest of the paddle is the ball. if the ball enter the green region at the bottom, the ball is lost. Add small amounts of perturbation (a value between 0 and 0.00001) to the velocity in the x and the y components. Without this perturbation, if the ball moves horizontally at the center of playground and there is no brick along this horizontal line (unlikely, but possible), the ball will never change the direction.
- Bricks: They are at the middle of the playground. Each brick is a circle. The bricks may have different radii. There should be at least 1 pixel gap between bricks. The actual sizes of bricks and gaps are left for your decision. There should be at least 15 bricks when the game starts. When a brick is hit by the ball, the brick is eliminated.
- Menu: There is a menubar and a menu. The menu contains at least four menu items: Demo, Play, Save, and Open.
- Demo mode: the paddle automatically tracks the movement of the ball and reflects the ball back to the playground at all times. The game is not reponsive to mouse or keyboard in this mode. Menuitems 'Save' and 'Open' are grayed and disabled.
- Play mode: a human player can move the paddle by moving the mouse horizontally or pressing the left/right arrow keys. The paddle must not exceed the boundary of the white background. Menuitems 'Save' and 'Open' are enabled.
- Save: a FileChooser dialog appears and asks user to provide a file name. The current status of the game is saved in the file. Click here for details about Java's FileChooser.
- Open: a FileChooser dialog appears and asks user to provide a file name. Then the game status is restored from the saved file.
The game is frozen (i.e. the ball does not move) when the menu is clicked. You are encouraged to add more menu items as part of the program development, for example, one menu item to test the reflection of the ball when it hits the paddle.
- Status bar: The status bar shows one line of message at the bottom of the window. It can be any string (e.g. 'Welcome to Breakout Game'), plus the current position of the ball, in the (x,y) format using pixel as unit.
- Suppose all surface are rigid, i.e. their shapes do not change during collisions.
Programming Environment in MSEE 190
Several programming tools are installed and available for you to use. For details, please read /home/shay/a/sfwtools/public/README. If in doubt, type 'java -version' and 'qmake -version' in a terminal. The correct version for Java is '1.6.0_02' and '4.4.3' for QT. You can also manually set the correct path to enable these tools in case the settings are not properly applied. If you use tcsh, you can simply add the following line
source /home/shay/a/sfwtools/public/settings
in your .cshrc. You need to use an ee462xxx account to execute these programs. This account is given to you through Blackboard. Do not use your personal Purdue account as some tools are available to ee462xxx accounts only.
Program Functionality (4pt)
- (0.5pt) A main window appears after the program is launched. The window contains (at least) a canvas (i.e. playground, where the game is rendered), a status bar at the bottom and a menubar containing four menu items (Demo, Play, Save, and Open).
- (0.5pt) Black background, white playground, at least 15 circle bricks, a ball and a semicircular paddle is properly drawn in the canvas.
- (0.5pt) The ball bounces correctly when hitting bricks, paddle and boundary of the playground (following law of reflection).
- (0.5pt) Human player can control the right paddle by mouse movement and keyboard (left/right arrow keys) in Play Mode. The paddle should not go beyond the boundary of the playground.
- (0.2pt) The status bar tracks the position of the ball.
- (0.3pt) Game is over when all bricks are removed or the ball is lost. A message box pops up to show the result (win or lose).
- (0.3pt) When Save menu is clicked, a Save dialog appears. After the user chooses a file name, the game status is saved.
- (0.5pt) When Open menu is clicked, an Open dialog appears. After the user specifies a file, the saved status is restored. Save and Open functions are graded together. The functions are tested in the way that a status is first saved and then opened. You can receive the points for Open only if you have implemented Save correctly.
- (0.5pt) In Demo mode, the paddle moves automatically to catch the ball. A message is shown on the playground indicating the Demo mode. Human player cannot control the paddle meanwhile.
- (0.2pt) The game is frozen when a menu is selected and resumed when the menu is deactivated. The game is initialized when mode is changed, and switching between Play and Demo modes works properly.
Program Readability (1pt)
Coding Style (0.5pt)
- (0.1pt) The code is formatted uniformly with proper indentation. Make your code look beautiful and professional. Use code formatter in your IDE (both NetBeans and Eclipse provide such function).
- (0.2pt) Classes, objects and members are named under coding conventions. There are several conventions for your choice to follow:
As there are many existing conventions for C++ coding, the principle of your coding style is to be consistent, understandable and easy to read.
- (0.2pt) There should be explanatory comments to help reader understand your code. Also, give a brief description about the class at the beginning of each class file.
Documentation (0.5pt)
- (0.3pt) Explain what classes and objects are created for this program. State the purpose of inheriting from library classes (e.g. JWindow or QMainWindow). Demonstrate your understanding of OO concepts such as inheritance and polymorphism that are applied in your program.
- (0.1pt) Analyze the collision detection algorithm and explain the implementation of bouncing and reflection.
- (0.1pt) Explain the structure of the status files for Save and Open functionality.
Please list everything you have implemented in your documentation. If something is implemented in the source code but not mentioned in your documentation, it is not graded and you will not receive any point.
Your documentations can be in one of two formats (1) PDF or (2) README. A Word file is not accepted because there are too many versions of Word programs and the formatting can be different. README is an ASCII file and the name must be README. This is a widely accepted convention by millions of people. The file name is not readme, readme.txt, Readme, or anything else. It must be README.
Avoid plagiarism: Whenever you cite text, pictures, ideas, etc., be sure you include the source in your reference. This includes information from the Internet.
Bonus (3 pt)
- (0.5pt) Use of sound.
- (0.5pt) Make it an applet.
- (1pt). The demo mode controls the angles of collisions so that the ball always bounces directly back to the paddle without hitting to the wall. You will receive 0.5 point for the design and another 0.5 point for the implementation. Please note that you have accomplished this bonus task in your documentation.
- (1pt). Given the initial location and the velocity of the ball and the locations of the bricks (up to 4), show whether it is possible for the paddle to hit the ball once and eliminate all bricks without hitting the paddle again. Hitting the wall is alllowed.
Submission Procedure
- Pack your code: put your source code in a CVS repository (see how) and make a zip file of the repository using the following command:
zip -r ipa1.zip <repository_dir>where <repository_dir> is the subdirectory of your CVS repository for this assignment.
- Verify the zip file: open the zip file and verify that the names of the content files have trailing ",v" (comma v). This means that your submission is a package of CVS repository, not the unmanaged source files. If you do not submit CVS repository files, you will get zero for the assignment.
- Prepare your documentation file: acceptable formats of documentation files are PDF and README. If you use PDF, make sure your document can be rendered correctly in Linux environment. If you write ASCII documentation file, name it as README (anything else could result in grade penalty).
- Upload the zip file and the documentation file as two attachments on Blackboard. Make sure they are uploaded to the correct assignment slot.
- Download the files and double check they are not corrupted or mistakenly uploaded.
Submission of assignments is controlled by Blackboard system. If you fail to submit the correct files to the correct slot, you can always withdraw your submission until the final deadline. You cannot correct your submissions after the final due date, and your assignments are graded based on what is left on Blackboard system. No email submissions/corrections will be accepted.
Only CVS repositories will be accepted in this course. SVN repositories are not acceptable.
Supplementary Materials
Computer Coordinates: If you have not written any program using graphics, you may not know that in a program, the origin is at the left upper corner, as shown below.
Law of Reflection: Suppose Vi is the ball’s original direction and N is the normal vector. The ball’s new direction is Vo. The angle between N and Vi is called the angle of incidence alpha ; the angle between N and Vo is called the angle of reflection beta. The law says that alpha = beta.
Normal Vector of Paddle: It is easy to calculate the normal vector of the paddel. It is the direction from the arc’s center C to the location of collision.
How to calculate the reflected direction Vo?
The sum of Vo and (-Vi) is a vector in the same direction as N. Therefore, we can write
Vo - Vi = sN,
here s is twice Vi's projection on N. In other words, s is twice the inner product between (-Vi) and N, namely 2 (-Vi) N.
Vo = Vi - 2 (Vi N) N
Let Vi = (xi, yi), N = (xn, yn), and Vo = (xo, yo).
xo = xi - 2 (xi xn + yi yn) xn
yo = yi - 2 (xi xn + yi yn) yn.
It would make the calculation easier if you keep the velocity and the normal vectors unit vectors.
Java fillArc

gfx.setColor(Color.BLACK);
gfx.fillArc(10, 10, 80, 80, 0, 90);
gfx.setColor(Color.RED);
gfx.fillArc(100, 10, 80, 80, 60, 60);
gfx.setColor(Color.GREEN);
gfx.fillArc(200, 10, 80, 80, 90, 180);
gfx.setColor(Color.BLUE);
gfx.fillArc(300, 10, 80, 80, 330, 60);
gfx.setColor(Color.YELLOW);
gfx.fillArc(10, 210, 80, 80, 150, 120);
gfx.setColor(Color.GRAY);
gfx.fillArc(100, 210, 80, 80, 270, 60);
gfx.setColor(Color.CYAN);
gfx.fillArc(200, 210, 80, 80, 0, 120);
gfx.setColor(Color.PINK);
gfx.fillArc(300, 210, 80, 80, 330, 180);
You can see a figure explaining the definition of the parametes here. You can find the document.
Java Arc2D
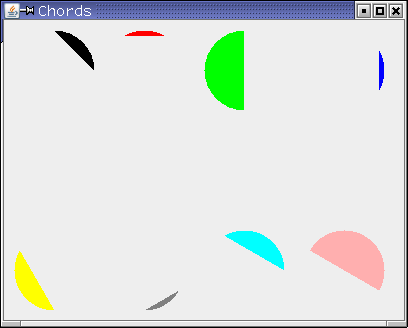
public void paintComponent(Graphics gfx) {
Graphics2D g2d = (Graphics2D) gfx;
gfx.setColor(Color.BLACK);
Arc2D ch1 = new Arc2D.Double();
ch1.setArc(10, 10, 80, 80, 0, 90, Arc2D.CHORD);
g2d.fill(ch1);
gfx.setColor(Color.RED);
Arc2D ch2 = new Arc2D.Double();
ch2.setArc(100, 10, 80, 80, 60, 60, Arc2D.CHORD);
g2d.fill(ch2);
gfx.setColor(Color.GREEN);
Arc2D ch3 = new Arc2D.Double();
ch3.setArc(200, 10, 80, 80, 90, 180, Arc2D.CHORD);
g2d.fill(ch3);
gfx.setColor(Color.BLUE);
Arc2D ch4 = new Arc2D.Double();
ch4.setArc(300, 10, 80, 80, 330, 60, Arc2D.CHORD);
g2d.fill(ch4);
gfx.setColor(Color.YELLOW);
Arc2D ch5 = new Arc2D.Double();
ch5.setArc(10, 210, 80, 80, 150, 120, Arc2D.CHORD);
g2d.fill(ch5);
gfx.setColor(Color.GRAY);
Arc2D ch6 = new Arc2D.Double();
ch6.setArc(100, 210, 80, 80, 270, 60, Arc2D.CHORD);
g2d.fill(ch6);
gfx.setColor(Color.CYAN);
Arc2D ch7 = new Arc2D.Double();
ch7.setArc(200, 210, 80, 80, 0, 120, Arc2D.CHORD);
g2d.fill(ch7);
gfx.setColor(Color.PINK);
Arc2D ch8 = new Arc2D.Double();
ch8.setArc(300, 210, 80, 80, 330, 180, Arc2D.CHORD);
g2d.fill(ch8);
}
The document of Java Arc2D is here.
Maintain Readable Source Codes: It is crucial to keep the readability of the source codes in a large-scale software project. Documentations and programming comments are two extremely important supports for program readability. Therefore, some relevant requirements are made in the assignment. You may want to check the following websites to have a brief idea of how to maintain qualified documentations, comments and code styles.
|