Assignment Notes
ENGR 132
Need access to Gradescope?
- Log into Brightspace and open your ENGR 132 course.
- Click Content from the black menu ribbon at the top of the page.
- Click Gradescope from the Table of Contents in the left sidebar.
- Click the Gradescope link, which will open your section’s Gradescope course.
- Select the assignment you are ready to submit.
Be careful to name your deliverables correctly before you submit them. Submit all the files requested. Do not include files not listed in the deliverables.
Opening Gradescope through Brightspace will auto-enroll you in the Gradescope course for your section. You can access Gradescope through Brightspace throughout the semester.
M-file vs Live Code in MATLAB
You must use m-files for this course. You can confirm your submission is the correct type if it has the *.m extension. Live files with the .mlx extension will not be accepted.
Document, Test, Debug, and Finalize Your Code
- Comment your code while you are coding, not afterwards. It is easy to forget what each line of code represents if you delay commenting and waiting until the end to add comments increases the time you will spend on commenting.
- Re-save, run, and debug your code often, preferably after each new line or closely related 2-5 new lines of code are added. This allows you to identify the true location of problems more easily. MATLAB identifies the first line of code that fails, but the actual error could be on any previous line.
- Suppress printing of code that is functioning properly. Only formatted displays should be printed in the Command Window once your code is functional.
- Ensure your script will run when the Workspace is empty. A properly functioning script should contain all the necessary commands within the script itself. Be careful if you test commands or store variables in the Workspace that are not created from the script. To test your final code, clear the Workspace completely and then run the script from the command prompt. Only variables created by your script should remain in the Workspace and no errors appear in the Command Window.
Creating Plots of Data
- When analyzing bivariate (two variables) data, you must determine which is the independent variable and which is the dependent variable.
- A common way to phrase a request for a plot is to say, “Plot variable 1 versus variable 2.” Variable 1 refers to the y-axis variable; variable 2 refers to the x-axis variable.
- Be sure to use the proper line and/or marker style for this class.
Creating Plots of Models
- When plotting models, use lines with no data markers. The points used to generate the plot are selected for convenience and do not refer to actual data.
- MATLAB plots discrete points on a graph: a line is created by connecting the points. You want a smooth line when you plot a model, so you must have enough points in the independent variable vector when you calculate the dependent variable vector. Having enough points allows curved lines to display smoothly. Note that the number of points necessary to make a smooth model line can vary, depending on the data set. A good rule of thumb for this course is to create with an x-vector that starts with the lowest x data value, ends with the largest x data value, and has 50 points. If it doesn’t appear smooth with 50 points, increase the number of points until the line does appear smooth.
- You can present a model with its raw data on the same plot. The model is a line with no data markers. The raw data are data markers with no connecting line.
Testing and Debugging Plot Code
- Always close all figure windows before re-running your code. Otherwise, your code will add or remove things from the existing plot displays. This can appear randomly in ways that may or may not reflect the presence of any coding problems.
The ‘find’ Command in the MATLAB Editor
If you use the find
command within the MATLAB editor, you may notice that MATLAB sometimes produces a warning on the lines with the find command. MATLAB may suggest that you use logical indexing, which allows you to use logical 1s and 0s to identify which values in a vector correspond to the ‘true’ condition, similar to array indexing. You can use either method (find
or logical indexing) when appropriate. There may be times where only one of them works. You should know how the find
command works for exams and quizzes.
Intervals and Ranges
A range of numbers can be defined as an interval. If a boundary value is included within the range, then we say the range is “inclusive” of the number. If a boundary value is not included in the range, then the range is “exclusive” (or not inclusive) of that number. See examples below for the range of number 5 to 10.
Relational notation | Interval notation | Explanation |
---|---|---|
5 ≤ x ≤ 10 | [5, 10] | The numbers between 5 and 10, inclusive of both 5 and 10 |
5 ≤ x < 10 | [5, 10) | The numbers between 5 and 10, inclusive of only 5 (exclusive of 10) |
5 < x ≤ 10 | (5, 10] | The numbers between 5 and 10, inclusive of only 10 (exclusive of 5) |
5 < x < 10 | (5, 10) | The numbers between 5 and 10, exclusive of both 5 and 10 |
Coding Long Expressions
Coding large expressions or commands in one line is difficult. For example, you need to code one solution to a quadratic equation using the formula
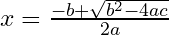
using variables a, b, and c.
You have two options to manage statement length.
Build from smaller terms
Calculate smaller terms that you assign to MATLAB variables, then build the expression from the assigned variables and any remaining terms.
% Find x using the quadratic formula
discrim = sqrt(b^2 – 4*a*c);
denom = 2*a;
x = (-b + discrim)/denom;
Use the MATLAB ellipsis
Use MATLAB’s ellipsis (…) to break up long lines of code for readability. Read this MATLAB documentation for more guidance on using the ellipsis: Continue Long Statements on Multiple Lines
% Find x using the quadratic formula
x = (-b + sqrt(b^2 - 4*a*c)) ...
/(2*a);
String Arrays in MATLAB
MATLAB allows you to create a string array, which is an array where each element in the array is a string instead of a number. It is important that you use proper formatting in a string array. Enter this command into MATLAB:
purdue_astronauts = ["Neil Armstrong","Eugene Cernan","Loral OHara","Scott Tingle"]
The double quotes are required; single quotes will not create a string array. You can use array commands, such as numel
and size
, on string arrays.