Due 4/7
qsort
Goals
The goals of this assignment are as follows:
- Learn to use function addresses
(function pointers). - Learn to use the
qsort(…)
function. - Gain some perspective on different sorting algorithms.
Overview
This homework is about sorting.
For the more practical approach, you will also write a wrapper function for the standard
qsort(…)
function, an implementation of the
quicksort algorithm.
There is one starter file: sorts.h. To get it, run 264get ec01
Outline of a solution
👌 Okay to copy/adapt anything you see in these two screenshot images. (You may not copy code from lecture or anywhere else.)
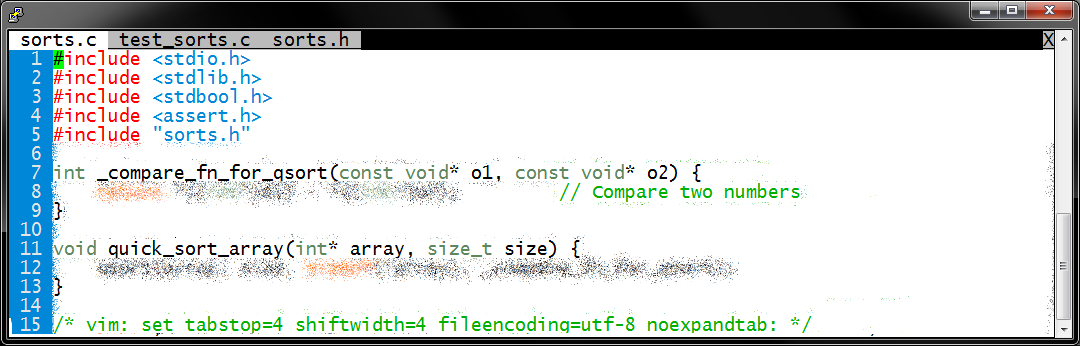
Demonstration
Reminder: Do not copy this code or anything else into your code (unless marked as
"Okay to copy/adapt" by one of the instructors). However, you may run this in your account
by running
264get EC01
.
Output
Before quick_sort_array(array2) 5 4 2 1 7 6 3 After quick_sort_array(array2) 1 2 3 4 5 6 7
Requirements
- Your submission must contain each of the following files, as specified:
- This should simply call the
qsort(…)
library function. - The
qsort(…)
function requires the use of function addresses (function pointers). - This may not result in any heap allocation (i.e., calls to
malloc(…)
) by your code. - Yes, this is easy, but make sure you understand how it works!
- This must cause every line of code in your sorts.c to be executed.
- Every public function in sorts.c must be called directly from
main(…)
and/or from a helper within test_sorts.c. - Only the following externally defined functions and constants are allowed in your .c files. (You may put the corresponding #include <…> statements in the .c file or in your sorts.h, at your option.)
header functions/symbols allowed in… stdbool.h bool
,true
,false
sorts.c
,sorts.h
,test_sorts.c
assert.h assert
sorts.c
,test_sorts.c
stdio.h printf
,fprintf
,stdout
,FILE
test_sorts.c
stdlib.h malloc
,free
,NULL
,size_t
,EXIT_SUCCESS
,EXIT_FAILURE
sorts.c
,test_sorts.c
,sorts.h
- Submissions must meet the code quality standards and the policies on homework and academic integrity.
file | contents | |
---|---|---|
sorts.c | function definitions |
quick sort array(int✶ array, size t size)
→ return type: void
Sort array using the qsort(…) standard library function.
|
test_sorts.c | function definitions |
main(int argc, char✶ argv[])
→ return type: int
Test your functions in sorts.c.
|
Submit
To submit EC01 from within your ec01 directory,
type
264submit EC01 sorts.h sorts.c test_sorts.c expected.txt miniunit.h clog.h Makefile
Pre-tester ●
The pre-tester for EC01 has not yet been released. As soon as it is ready, this note will be changed.
Q&A
-
Can I add helper functions to sorts.c?
Yes. Make sure the names begin with "_". Also, they should be declared as static. -
Is it a violation of the spec if
qsort(…)
callsmalloc(…)
?
No. The only requirement is that your code not callmalloc(…)
as a result of callingquick_sort_array(…)
.
Updates
4/4/2022 |
|