Images (A)
Goals
- Learn how to program with binary files
- Practice using structures
Overview
In this exercise, you will write code to read, write, and crop BMP image files.
The BMP file format
A BMP file has the following format:
Header | 54 bytes |
Palette (optional) | 0 bytes (for 24-bit RGB images) |
Image Data | file size - 54 (for 24-bit RGB images) |
The header has 54 bytes, which are divided into the following fields. Note that the #pragma directive ensures that the header structure is really 54-byte long by using 1-byte alignment.
typedef struct { // Total: 54 bytes uint16_t type; // Magic identifier: 0x4d42 uint32_t size; // File size in bytes uint16_t reserved1; // Not used uint16_t reserved2; // Not used uint32_t offset; // Offset to image data in bytes from beginning of file (54 bytes) uint32_t dib_header_size; // DIB Header size in bytes (40 bytes) int32_t width_px; // Width of the image int32_t height_px; // Height of image uint16_t num_planes; // Number of color planes uint16_t bits_per_pixel; // Bits per pixel uint32_t compression; // Compression type uint32_t image_size_bytes; // Image size in bytes int32_t x_resolution_ppm; // Pixels per meter int32_t y_resolution_ppm; // Pixels per meter uint32_t num_colors; // Number of colors uint32_t important_colors; // Important colors } BMPHeader;
Note that the number of bytes each field occupies can be obtained by dividing the number 16 or 32 by 8. For example, the field "type" occupies 2 bytes. These fields are all integers. An "uint" means unsigned, and "int" means signed. For example the fields "width" and "height" are signed integers. However, for simplicity, all the BMP files we have will contain only positive integers. You may assume that in your code. Also, we are dealing wih uncompressed BMP format (compression field is 0).
Because of the packing specified in the bmp.h file, you should be able to use fread to read in the first 54 bytes of a BMP file and store 54 bytes in a BMPHeader structure.
Among all these fields in the BMPHeader structure, you have to pay attention to the following fields:
bits | number of bits per pixel |
width | number of pixel per row |
height | number of rows |
size | file size |
imagesize | the size of image data (file size - size of header, which is 54) |
We will further explain bits, width, height, and imagesize later. You should use the following structure to store a BMP file, the header for the first 54 bytes of a given BMP file, and data should point to a location that is big enough (of imagesize) to store the image data (color information of each pixel).
typedef struct { BMPHeader header; unsigned char* data; } BMPImage;
Effectively, the BMPImage structure stores the entire BMP file.
Now, let's examine the fields bits, width, height, and imagesize in
greater details. The bits field records the number of bits used to
represent a pixel. For this assignment
exercise (and the next exercise and assignment),
we are dealing with BMP files with only 24 bits per pixel or 16 bits per pixel.
For 24-bit representation, 8 bits (1 byte) for RED, 8 bits for GREEN, and 8 bits
for BLUE.
For 16-bit representation, each color is represented using 5 bits
(the most significant bit is not used). For this exercise, we will use
only 24-bit BMP files to test your functions. However, your code should be
able to handle 16-bit format as well. (Note that the header format is
actually more complicated for 16-bit format. However, for this exercise
and the next exercise and assignment, we will use the same header format
for both 24-bit and 16-bit BMP files for simplicity. So yes, we are abusing
the format!)
The width field gives you the number of pixels per row. Therefore, the total number of bytes required to represent a row of pixel for a 24-bit representation is width * 3. However, the BMP format requires each row to be padded at the end such that each row is represented by multiples of 4 bytes of data. For example, if there is only one pixel in each row, we need an additional byte to pad a row. If there are two pixels per row, 2 additional bytes. If there are three pixels per row, 3 additional bytes. If there are four pixels per row, we don't have too perform padding. We require you to assign value 0 to each of the padding byte.
The height field gives you the number of rows. Row 0 is the bottom of the image. The file is organized such that the bottom row follows the header, and the top row is at the end of the file. Within each row, the left most pixel has a lower index. Therefore, the first byte in data, i.e., data[0], belongs to the bottom left pixel.
The imagesize field is height * amount of data per row. Note that the amount of date per row includes padding at the end of each row.
You can visualize the one-dimensional data as a three-dimensional array,
which is organized as rows of pixels, with each pixel represented by 3 bytes
of colors (24-bit representation) or 2 bytes of colors (16-bit representation).
However, because of padding, you cannot easily typecast the one-dimensional
data as a 3-dimensional array. Instead, you can first typecast it
as a two dimensional array, rows of pixels. For each row of data, you
can typecast it as a two-dimensional array, where the first dimension
captures pixels from left to right, the second dimension is the color
of each pixel (3 bytes or 2 bytes).
The Wikipedia article on the BMP file format has a nice diagram and more complete details about this format.
Examining a BMP file directly
The best way to inspect binary data is with a hex dump. From bash, you can type xxd myimage.bmp
. Since it will probably be long, you will want to view in vim. One way to do that is type xxd myimage.bmp | vim -
from bash. Another way is to open the file in vim and then type :%!xxd
. (Do not save!)
Suppose you have the following tiny 6x6 BMP image: . (Yes, it really is only 6 pixels by 6 pixels. Don't worry. A larger version is included in one of the diagrams below.)
To get a hex dump right on the command line, you could type this at bash:
$ xxd 6x6_24bit.bmp
It will be more convenient to view in vim, so we type this from bash instead. (Don't forget the "-" at the end!)
$ xxd 6x6_24bit.bmp | vim -
Here is the hex dump, a you will see it. Don't worry if this looks cryptic. Read on and you will understand it completely.
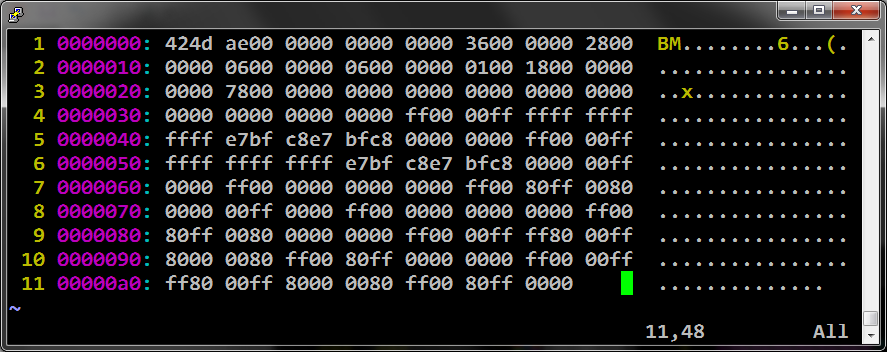
You can break this apart using the BMP header specification (copied from above).
typedef struct { // Total: 54 bytes uint16_t type; // Magic identifier: 0x4d42 uint32_t size; // File size in bytes uint16_t reserved1; // Not used uint16_t reserved2; // Not used uint32_t offset; // Offset to image data in bytes from beginning of file (54 bytes) uint32_t dib_header_size; // DIB Header size in bytes (40 bytes) int32_t width_px; // Width of the image int32_t height_px; // Height of image uint16_t num_planes; // Number of color planes uint16_t bits_per_pixel; // Bits per pixel uint32_t compression; // Compression type uint32_t image_size_bytes; // Image size in bytes int32_t x_resolution_ppm; // Pixels per meter int32_t y_resolution_ppm; // Pixels per meter uint32_t num_colors; // Number of colors uint32_t important_colors; // Important colors } BMPHeader;
Here is the same hex dump, this time with some annotations.
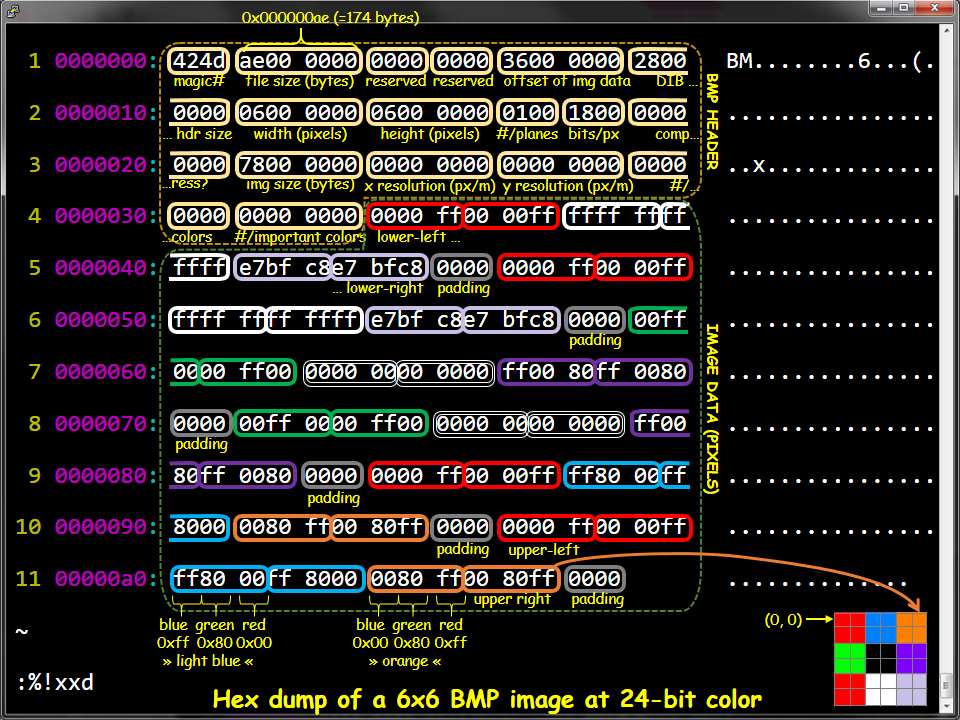
For this and other binary file formats, you can understand what value goes where by simply looking at the specification and a hex dump of the binary file.
Big-endian vs. little-endian
You may have noticed in the hex dump of the image that the file size (174) is represented as “ae00 0000” (instead of “0000 00ae”). This is because the BMP format is a little endian format. In short, that means the number 0x12345678 (305419896 in decimal notation) will be stored in memory as 0x78 0x56 0x34 0x12.
Remember that two hex digits are one byte. For example, 0x12345678 consist of four bytes: 0x12, 0x34, 0x56, and 0x78. When we store it using little endian, we store the least significant byte (LSB) first in the file (or memory). For that reason, little endian can also be called LSB first.
This may seem counter-intuitive because our own writing system in the physical world is the opposite: big endian. Thus, if you write, “I have 57 pints of ice cream in my freezer,” 5 is the most significant digit of 57, and we write it first.
Little endian architectures include Intel x86 and x86-64 (used on desktop computers and most laptops), as well as most ARM-based CPUs used in phones and tablets. Notably, ecegrid (x86-64) uses little-endian.
Big endian architectures are harder to find, and mostly confined to very old architectures (e.g., IBM System/360, Motorola 68000-series) and relatively exotic server architectures that are based on those old ones (e.g., IBM z/Architecture, Freescale ColdFire).
Important: Endianness only pertains to the order in which the individual bytes
comprising multi-byte numeric types (e.g., int
,
long
, etc.) are stored. It does not affect the order of
individual bits within a byte, or the elements in normal arrays.
See the Q&A for more.
Checking your understanding
To check your understanding of the file format, try asking yourself the following questions:
- How big is this BMP image (in terms of pixels)?
- How many bytes is the file?
- For the BMP header:
- How many bytes does it take up?
- Where in the diagram is it?
- What is its file offset (i.e., number of bytes from the start of the file)?
- Consider the pixel in the lower-left corner of the image.
- What color is it?
- Where is the data (3 bytes) in the diagram?
- How many bytes is that from the beginning of the image data (pixels)?
- How many bytes is that from the beginning of the file?
- What are its (x,y) coordinates? (Hint: See the small representation of the image in the lower-right corner of that diagram.)
- Consider the pixel in the upper-right corner. … and finally the lower-left corner. Ask yourself the same questions about each of those pixels.
- Find some "padding" in the diagram. How many bytes is it?
- How many bytes does a single row of pixels take up in this image (including the padding)?
- Will the number of padding bytes per row always be the same within a given image?
- … for all BMP images?
- For an arbitrary image that is w pixels wide, at what file offset will the ith row begin?
Handling run-time errors
In this assignment, you will need to handle run-time errors. These are not the same as bugs in your code. These are problems (or special conditions) that might arise due to the inputs from the caller of a function that you write. For example, a file may be inaccessible or corrupt, or
malloc(…)
may fail and return NULL.
For purposes of this assignment, the error-handling strategy will be two-pronged:
- Return a special value if the operation failed. For functions that return a
FILE*
, you will return NULL if the operation failed. - Return an error message via pass-by-address. Normally, the caller will pass the address of
char*
. If the operation is successful, the callee will do nothing with it. However, if there is a failure, the callee will return a newly heap-allocated string. It is the caller's responsibility to free it.
You will do this for all functions in this assignment that take a parameter called error
.
Here is a sketch of the basic pattern we are describing:
#include <stdio.h> #include <stdlib.h> #include <stdbool.h> #include <assert.h> bool do_something(int a, int b, char** error) { // ... if(success == false) { if(*error == NULL) { char* message = "do_something(..) failed because success == false"; *error = malloc((strlen(message) + 1) * sizeof(**error)); strcpy(*error, message); } return false; } // ... return true; } int main(int argc, char* argv[]) { char* error = NULL; bool do_something_succeeded = do_something(10, 11, &error); if(! do_something_succeeded) { fprintf(stderr, error); assert(error != NULL); free(error); return EXIT_FAILURE; } else { assert(error == NULL); } return EXIT_SUCCESS; }
Warm-up exercises
This assignment includes a warm-up exercise to help you get ready. This accounts for 10% of your score for HW15. Scoring will be relatively light, but the usual base requirements apply.
-
Read a text file
Create a function that reads the contents of a file and returns it as a string on the heap. The caller is responsible for freeing that memory. Usefopen(…)
,fread(…)
, andfclose(…)
. -
Write a text file
Create a function that writes the given content to a file at the specified path. Usefopen(…)
,fwrite(…)
, andfclose(…)
. -
Write a Point to a binary file
Write a function that writes a single Point to a binary file at the specified path. Usefopen(…)
,fwrite(…)
, andfclose(…)
. For thePoint
class, please copy-paste the following struct type into your file:typedef struct { int x; int y; } Point;
This will be a binary file. This is preparation for working with binary files for images, which work the same way. In general, you will usefwrite(…)
for binary files. -
Read a Point from a binary file
Create a function that reads aPoint
from the binary file at the specified path into aPoint
on the stack. Nomalloc(…)
orfree(…)
are necessary for this one. Usefopen(…)
,fread(…)
, andfclose(…)
.
The structure of the warmup.c file is described in the Requirements table below. You should write your own warmup.c. You may add helper functions, if you wish.
Opt out.
In a hurry, and don't need the practice? This warm-up is here to help you learn what you need to succeed on the rest of this assignment—not to add additional work. Therefore, we give you an option. Those who feel that they do not need the practice may "opt out". by modifying warmup.c so that it does nothing but print the following message exactly and then exit:
I already know this and do not need to practice.
If you do that, then your score for HW15 will be based solely on the rest of this assignment. If you leave the warmup.c undone, if you do not turn in a warmup.c, or if the message it prints does not match perfectly, then you will receive 0 for the warmup portion of this assignment (10%).
bmp.h and test files
We have provided a bmp.h file and some test image files. You must use our bmp.h. To obtain these, run 264get hw15
. You may use other BMP image files of your choice*, but not all BMP files will work with this code (e.g., grayscale, other color depths, etc.), so your may wish to stick with the supplied files to test.
Any image files that you turn in must be G-rated and not violate any copyrights (i.e., your own images or else freely licensed). Provide credit in your bmp.c file in the form of a comment like:
// Credit: blahblah.bmp, Fetty Wap, http://fettywap.com/free_photos/blahblah.bmp
Requirements
In all functions that accept
FILE* fp
, you may assume that it is valid and open in the correct mode. However, you may not assume anything about the amount of data or the position of the file pointer. Also, you may not assume that every call tofread(…)
orfwrite(…)
will succeed.In all functions that accept
char** error
, you must handle run-time errors as described in the section above (Handling run-time errors).
- Your submission must contain each of the following files, as specified:
- Return the image as a
BMPImage
on the heap. - Use your
check_bmp_header(…)
to check the integrity of the file. -
Handle all kinds of run-time errors using the method described above.
-
That means in case of an error, set
*error
to the address of a descriptive message on the heap and returnNULL
. - It is the caller's responsibility to free the memory for the error message.
-
That means in case of an error, set
- Do not leak memory under any circumstances.
-
Do not attempt to open or
close the file. It is already open.
-
fopen(…)
andfclose(…)
are not allowed in bmp.c. (See the table of allowed functions/symbols below.)
-
- Return true if and only if the given
BMPHeader
is valid. - A header is valid if
① its magic number is 0x4d42,
② image data begins immediately after the header data
(
header -> offset == BMP HEADER SIZE
), ③ the DIB header is the correct size (DIB_HEADER_SIZE
), ④ there is only one image plane, ⑤ there is no compression (header->compression == 0
), ⑥num_colors
andimportant_colors
are both 0, ⑦ the image has either16 or24 bits per pixel, ⑧ thesize
andimage_size_bytes
fields are correct in relation to thebits
,width
, andheight
fieldsorand in relation to the file size. -
Hints: Q28 and Q29 offer some optional suggestions on how you can
structure this, and the relation to
read_bmp(…)
- Return true if and only if the operation succeeded.
- Handle run-time errors using the method described above.
-
That means in case of an error, set
*error
to the address of a descriptive message on the heap and returnfalse
. - It is the caller's responsibility to free the memory for the error message.
-
That means in case of an error, set
- This should not close the file.
- Do not leak memory under any circumstances.
-
Do not attempt to open or close the file. It is already open.
-
fopen(…)
andfclose(…)
are not allowed in bmp.c. (See the table of allowed functions/symbols below.)
-
- You may assume the file is initially empty.
- x is the start index, from the left edge of the input image.
- y is the start index, from the top edge of the input image.
- w is the width of the new image.
- h is the height of the new image.
- This will create a new BMPImage, including a BMPHeader that reflects the width and height (and related fields) for the new image (w and h). Copy in pixel data from the original image into the new image data.
- Handle run-time errors using the method described above.
-
That means in case of an error, set
*error
to the address of a descriptive message on the heap and returnNULL
. - It is the caller's responsibility to free the memory for the error message.
-
Since
crop_bmp(…)
does not read or write any files, the error handling will be somewhat simpler here.
-
That means in case of an error, set
- Running your
main(…)
with no command line arguments should cover all of your code in bmp.c, except for the parts that deal with allocation failures. (You do need to test other kinds of errors.) - We should be able to compile and run your test_bmp.c with someone else's bmp.c.
- Your test should return EXIT_SUCCESS and produce the output in expected.txt if and only if the implementation code (bmp.c) is correct.
- If the bmp.c is incorrect, this should return
EXIT_FAILURE
and/or print output different from your expected.txt. - Your test should print some sort of human-readable output to stdout
(i.e., using
printf(…)
). - When your test_bmp.c runs, the output (on stdout) should be identical to the contents of this file, if and only if the bmp.c it was compiled with is correct.
- This can be anything you like, as long as it distinguishes a working implementation (bmp.c) from a flawed one.
- You will write this file by hand. (This is not created by your code.)
- To check if your output matches, run:
gcc bmp.c -o bmp diff <(./bmp) expected.txt(bmp is the name of your executable, not an image file.)To compare a lot of output more easily, you can use vimdiff instead of diff:vimdiff <(./bmp) expected.txt
- ⚠ This should consist of human-readable text—not an image file.
- The caller is responsible for freeing that memory.
- Use
fopen(…)
,fread(…)
, andfclose(…)
. - Use
fopen(…)
,fwrite(…)
, andfclose(…)
. - Use
fopen(…)
,fwrite(…)
, andfclose(…)
. - For the
Point
class, please copy-paste the following struct type into your file:typedef struct { int x; int y; } Point;
- You may assume the file can be written to successfully (i.e., no error handling).
- No
malloc(…)
orfree(…)
are necessary for this one. - Use
fopen(…)
,fread(…)
, andfclose(…)
. - You may assume the file can be read successfully (i.e., no error handling).
-
Only the following externally defined functions and constants are allowed in your .c files.
header functions/symbols allowed in… assert.h assert(…)
bmp.c
,test_bmp.c
,warmup.c
string.h strcat(…)
,strlen(…)
,strcpy(…)
,strcmp(…)
,strerror(…)
,strncpy(…)
,memcpy(…)
bmp.c
,test_bmp.c
,warmup.c
stdbool.h true
,false
bmp.c
,test_bmp.c
,warmup.c
stdlib.h malloc(…)
,free(…)
,NULL
,EXIT_SUCCESS
,EXIT_FAILURE
bmp.c
,test_bmp.c
,warmup.c
stdio.h clearerr(…)
,feof(…)
,ferror(…)
,fgetpos(…)
,FILE
,fread(…)
,fseek(…)
,ftell(…)
,ftello(…)
,fwrite(…)
,fflush(…
,EOF
,SEEK_CUR
,SEEK_SET
,SEEK_END
bmp.c
,test_bmp.c
,warmup.c
stdio.h fopen(…)
,fclose(…)
,printf(…)
,fprintf(…)
,stdout
test_bmp.c
,warmup.c
- Make no assumptions about the maximum image size.
-
No function in bmp.c except for
free_bmp(…)
may modify its arguments or any memory referred to directly or indirectly by its arguments. -
You may optionally include other BMP files (G-rated and non-copyright-infringing) that your test code uses.
- ⚠ Do not submit any of the starter images that we provided.
- ⚠ If you submit your own images, they must be <10,000 bytes.
- Do not modify the bmp.h file.
- All files must be in one directory. Do not put images in subdirectories.
- Submissions must meet the code quality standards and the policies on homework and academic integrity.
file | contents | |
---|---|---|
bmp.c | functions |
read bmp(FILE✶ fp, char✶✶ error)
→ return type: BMPImage✶
Read a BMP image from an already open file.
|
check bmp header(BMPHeader✶ bmp hdr, FILE✶ fp)
→ return type: bool
Test if the BMPHeader is consistent with itself and the already open image file.
|
||
write bmp(FILE✶ fp, BMPImage✶ image, char✶✶ error)
→ return type: bool
Write an image to an already open file.
|
||
free bmp(BMPImage✶ image)
→ return type: void
Free all memory referred to by the given BMPImage .
|
||
crop bmp(BMPImage✶ image, int x, int y, int w, int h, char✶✶ error)
→ return type: BMPImage✶
Create a new image containing the cropped portion of the given image.
|
||
test_bmp.c | function |
main(…)
→ return type: int
Test all of the required functions in your bmp.c.
|
expected.txt | output |
Expected output from running your test_bmp.c.
|
warmup.c | functions |
main(int argc, char✶ argv[])
return 0 at the end to avoid problems with our tester.)
|
read file(const char✶ path, char✶✶ error)
→ return type: char ✶
Reads the contents of a file and returns it as a string on the heap.
|
||
write file(const char✶ path, const char✶ contents, char✶✶ error)
→ return type: void
Write the given content to a file at the specified path.
|
||
write point(char✶ path, Point p, char✶✶ error)
→ return type: void
Writes a single Point to a file at the specified path.
|
||
read point(const char✶ path, char✶✶ error)
→ return type: Point
Read a Point from the file at the specified path into a Point on the stack.
|
Submit
To submit HW15, type
264submit HW15 bmp.c test_bmp.c expected.txt warmup.c
from inside your hw15 directory.
Pre-tester ●
The pre-tester for HW15 has been released and is ready to use.
Q&A
-
Do I need to manually reverse the bytes when I read/write a BMP file?
No, not on our platform.
For this assignment you are copying bytes directly from a file to memory, and back. Luckily for you, the x86 and x64 architectures, which power most Linux and Windows computers happen to use little-endian for storing numbers in memory. You may have noticed this when using thex/…
command in gcc.
In fact, the choice of little- or big-endian is actually a bit arbitrary, at least for fixed-width storage in memory or binary files. -
Are the bits reversed, too?
How would you know? … Remember, it's all just bytes. -
Would a string representation of an integer (e.g.,
char* s = "abc"
) also be reversed in memory?
No.
Long version: A string is just an array of characters, and each character is really just a number. Endian-ness only affects how a single number is stored, not bigger structures such as arrays or structures. Also, since achar
is only one byte on our platform (actually, all platforms by virtue of a special provision in the standard, but I digress...), endian-ness would not affect even an individual character because it only applies to the order of bytes within a number requiring multiple bytes to store in a file or memory.
You can observe this directly from gdb. -
Why are the RGB color components written in blue-green-red order (instead of red-blue-green)?
This also has to do with BMP being a little endian format. -
What are uint16_t and uint32_t?
These are special types that have a guaranteed size of 2 and 4 bytes, respectively. -
Do I still need to use
sizeof(…)
when referring to the size of uint16_t and uint32_t?
Yes. -
What is
unsigned
?
Theunsigned
keyword is a part of some type names (e.g., unsigned int, unsigned char, etc.) and indicates that the type cannot be negative. -
Are there other types I should know about?
“Should” is relative, but yeah, there are many other numeric types. Wikipedia has a decent list. -
Will all of this be on the exam?
All of the concepts in this and the other assignments can be considered within scope. That includes this Q&A. -
What does “valid” mean for
check_bmp_header(…)
?
You are checking that none of the information in the header contradicts itself or the contents of the file (esp. the file size). -
Is the gray_earth.bmp image valid?
Yes and no. That file follows the BMPv5 format specification. For this assignment we are following the simpler BMPv3 format specification. You may want to ignore that file. -
Can we use
assert(…)
in ourcheck_bmp_header(…)
?
Yes and no… but mostly no. You may useassert(…)
wherever you like, but only for detecting errors in your code; it should never be used to check for errors in the inputs or anything else. In other words, it is not for run-time checking.
Real-world software development operations typically use compiler features to effectively remove allassert(…)
statements prior to shipping a product. Thus, you should useassert(…)
only for things that need not be checked after the product is completed and deployed to users. -
Why do we need
ftell(…)
?
It might help you get the file size. (We'll leave it up to you to discover how, and why that would be needed in the first place.) -
Why does
check_bmp_header(…)
take aFILE* fp
as a parameter?
You should use that to make sure the actual file size matches the information in the BMP header. See Q15. -
Why does
fwrite(…)
add a newline character (0x0a)? to the end of my file
It doesn't. If you open the binary file in vim and then use:%!xxd
you may see an extraneous 0x0a at the end. That's because vim (like many code editors) adds a newline to the end of a file, if there's not one there already. Some solutions:
- (BEST) Use
xxd myfile.bmp | vim -
from bash. (Don't forget the '-' at the end!) - Ignore the 0x0a.
- Open the file with
vim -b myfile.bmp
and then use:%!xxd
to convert to the hex dump. The-b
tells vim to open it in binary mode, which (among other things) disables the newline at the end. - From within vim, open the file with
:tabe ++binary myfile.bmp
and then use:%!xxd
to convert to the hex dump. This also opens it in binary mode.
- (BEST) Use
-
How can
read_bmp(…)
return a descriptive error message whencheck_bmp_header(…)
does not?
You may want to use a helper function to do the checking for both. Your helper would be a lot likecheck_bmp_header(…)
but return an error message (i.e., via achar** error
). This isn't a requirement—just a suggestion. Use it only if you find it helpful. -
How are the pixels in a BMP numbered?
For purposes of the BMP image format, the pixels start in the lower-left, and progress left-to-right then bottom-to-top. See the diagram for a more concrete example.
For purposes of most image processing APIs and discussion, we generally designate (0,0) as the upper-left. -
What are some good helpers to use?
It's up to you. Here are some ideas to get you thinking:long int _get_file_size(…)
_Color _get_pixel(BMPImage* image, int x, int y)
typedef struct { unsigned char r, g, b; };
int _get_image_row_size_bytes(BMPHeader* bmp_hdr)
int _get_image_size_bytes(BMPHeader* bmp_hdr)
-
How do I read an image?
The structure of the BMP file format is given above under The BMP file format. In short, a BMP file consists of a header (BMPHeader
struct object stored in a binary file) plus an array ofunsigned char
(one byte each). Each pixel is three bytes, with one for each of red, green, and blue. You won't need to worry about the individual colors for this assignment.An example is shown in Q1 in this Q&A.The following explanation omits details about error checking.Yourread_file(…)
will first read aBMPHeader
from the file usingfread(…)
This is just like the warm-up. (An example shown in Prof. Quinn's lecture can be found on the schedule page.)Based on the information in theBMPHeader
object, you will know how many bytes are in the image pixel data. With that, you will read an array ofunsigned char
usingfread(…)
.Forfread(…)
, this is all you need. You won't need to do much with the pixel data, except forcrop_bmp(…)
. However, you do need to understand the fields in the image header in order to test it.The primary focus of this assignment is on testing. The reading and writing of images is not intended to be very challenging.Yourwrite_bmp(…)
will be very similar. It will write aBMPHeader
object usingfwrite(…)
and then usefwrite(…)
to write the pixel data. -
What should my error messages say?
There is no standard or required text. Just make sure the error message describes the problem specifically in a way that would make sense to a user. “Error” would be a poor error message. Examples of better error messages include “Unable to write to file”, “Corrupt: invalid padding between rows”, and so forth. -
How do the pieces of this file relate to the image?
Images are made up of small dots called "pixels". Images can be stored in a variety of ways. For this assignment, we focus on the BMP file format—and in particular, the 24-bit color format. In this format, each pixel consists of 3 bytes: red, green, and blue. This image data (pixels) is stored right after the header (54 bytes).The header is just a struct object containing many details about the image, such as the width, height, and file format. After the header, the pixels are laid out in a row in the file, starting with the lower-left pixel.Each row must be a multiple of 4 bytes. To ensure that is the case, 0 to 3 bytes of padding may be added to the end of each row.All of this is illustrated in the diagram (annotated xxd output) in Q1 above. Be sure you understand the diagram before you proceed. -
What is the value of the padding bytes?
The padding bytes are intentionally wasted space in the file. They are there to make the rows of pixel data line up. The value won't be used, but for consistency, they must contain zero. -
Is there any example code I can refer to?
This code from Prof. Lu's book is similar respects to this assignment. There are many differences between that code and this assignment, but you may find it useful to understand the high level. In particular, note how the error checking code is designed to prevent memory leaks, even when reading corrupt BMP files. Do not copy that code (or any other code). -
Should my program terminate from inside
read_bmp(…)
?
No. It should terminate only from the return statement at the end of yourmain(…)
. -
Should my
main(…)
take image file names from the command line (e.g., via argv)?
No. Hard code the image filenames in yourmain(…)
and include the image files with your submission. -
What if
read_file(…)
(or others) are called withNULL
for fp?
You may assume fp is notNULL
. -
What unit are x, y, width, and height measured in?
Pixels. -
Should
read_bmp(…)
callcheck_bmp_header(…)
?
You have freedom to design the implementations of these functions as you wish, but here is a hint. Since yourread_bmp(…)
needs to check the header and return an error message, you will probably want to make a helper function (check_bmp_header(BMPHeader* bmp_hdr, FILE* fp, char** error)
), which you would call from bothread_bmp(…)
and this function (check_bmp_header(…)
). but it will likely make -
How can I make the error checking code tighter, so it
doesn't obscure my program logic?
The snippets from Prof. Quinn's 12/4 lecture illustrate a way to structure your test code for this (and other) functions without obscuring the code logic. There are two small snippets, which you may use if you wish. See the schedule page. -
What does criteria ⑧ mean, under
check_bmp_header(…)
?
It says:the
In other words, you should be able to calculate thesize
andimage_size_bytes
fields are correct in relation to thebits
,width
, andheight
fieldsorand in relation to the file size..size
and.image_size_bytes
fields from the.bits
.width
, and.height
fields. Also, you should be able to calculate the file size from those fields. These should agree.
Updates
12/4/2017 | Removed references to 16-bit. Fixed a few trivial typos. |
12/7/2017 | Added Q28 and Q29. |