ECE 264 Advanced C Programming
IPA 3-1: Transformation of "Super" Blokus Pieces Representations
Due December 4, 2012 @ 6:00pm
Summary
This assignment asks you to build a program that can evaluate "Super" Blokus pieces, each with 3 - 10 squares. This is called "Super" Blokus because the original Blokus has pieces up to 5 squares only. so we extend the game to generate pieces containing 10 squares .
You need to design several algorithms to generate different pieces . Your algorithm should also detect duplicates.
In this assignment, all files use the ASCII (i.e. text) format.
"Super" Blokus Pieces
Tetris is a popular game that uses Blokus pieces. Tetris has 7 pieces, each with 4 squares, shown below.

as you can see Tetris allows mirror (the 'L' (blue) piece and the orange piece are mirrors and the 'z' (red) piece and the green piece are mirrors) . if we disallow mirrors then we get only 5 unique valid pieces made of 4 squares . Blokus considers mirrors duplicates so your Blokus algorithm should consider the mirrors as invalid piece.
The figure bellow shows the 5 valid pieces that blokus needs to generate when the number of squares is 4.
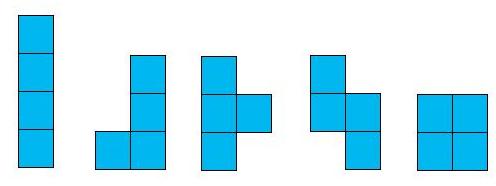
Blokus consider any rotation or mirroring of a piece as a duplicate and does not count it. The figure below shows the 8 different rotations and/or mirrors of a 4-square Blokus piece. Your algorithm should detect these as duplicates and generate only one of them

The following figure shows pieces of 6 squares.

Encoding
-
A piece is represented in the file using binary encoding using a string of 0's or 1's.
-
The length of the string is n2, here n is an integer between 4 and 10.
-
The ending character '\0' is not included in the n2 characters.
-
There are n 1's and the others are 0's.
The following is an example of a 5-squares piece encoding.

Stage 1 (5 points)
At this stage the program takes as input a file name (text file not binary). The file contains 1-d and 2-d representations of pieces. Your program should convert 1-d representations to 2-d representations and vice-versa. A 1-d representation stores the entire represenation on one line. For example, the piece
100100100
is a 1-D representation of a Blokus piece where a 1 represents a block and 0 represents an empty space. A 2-D representation displays the Blokus piece in a n X n format. For example, the piece
100
100
100
is a 2-D representation. A 1-D to 2-D transformation would take the piece
100100100
and transform it into
100
100
100
Each piece in the input files is separated by one blank line. For example, a file could look like
110100000
1000
1100
1000
0000
1100110000000000
Your output should be the converted pieces in the same order. Using the example from above, the output would be
110
100
000
1000110010000000
1100
1100
0000
0000
-
Testcases can contain combinations of 1-d and 2-d representations of pieces.
-
Error messages should be printed when the number of command-line arguments is not equal to 2 or if the input file cannot be opened and the program should exit with the status EXIT_FAILURE.
-
The biggest possible piece is 101 characters (100 characters for the actual blokus piece and 1 character for the following newline character).
-
Transformations should be printed in the same order they are in the input file.
-
You can find assignment file here. The zip file contains the following
-
ipa3_1.c - Skeleton for the main function
-
ipa3_1.h - Contains function headers and constants that can be useful for the assignment
-
utility.c - Contains the auxiliary functions for the assignment
-
Makefile - Can be used to compile and test assignment
-
Testcases and their corresponding solutions
-
You can edit anything in the .c files, the .h files, and the Makefile to fit your program.
WHAT YOU NEED TO SUBMIT?
1. All the .c and .h files needed to execute your assignment. All submissions for this IPA should have at least 2 .c files and 1 .h file. You can have more .c and .h files as you see fit. Any submissions with one .c file will have points deducted.
2. Makefile to compile your assignment. For each submission, the executable should be names ipa3_1.
3. A README file that describes the algorithm used in your assignment. The README should say how the functions accomplish their goals, not just the goals themselves.
HOW TO SUBMIT?
1. You can submit as many submission on the checking website as you would need. For this assignment, if you pass the testcases on the server, it will not guarantee a perfect score during grading.
2. Your submission for grading should be submitted through Blackboard. You can submit as many times on Blackboard as you need before the deadline. We will grade the final submission, so please make sure that it is your FINAL version with everything included.
HOW WILL WE GRADE YOUR SUBMISSION?
We will grade your assignments on algorithm correctness through DDD, memory allocation and deletions through valgrind , proper coding standards, content of README, a proper Makefile, and commenting.
|