ECE 462 Assignment 4
Pac-Man game using C++ and Qt (outcome 2)
In this assignment, you need to write a single player Pac-Man game. Your program must use C++ and Qt. If your assignment 2 does not pass outcome 2, this is the second and final chance.
Pac-Man is an interactive computer game developed in the early 1980s. It was one of the most popular games at that time. It is still being played by many people. The first World Championship was held in 2007 in New York City. You can play the game on-line in several web sites:

Game Components
The Pac-Man game has a menu and one widget on its main window -- the playfield, and everything should be drawn into the playfield. The necessary contents of the menu and playfield are listed as follows:
- Menu items: Menu items named "Reset", "Clear High Score","Exit" can respectively restart the game, reset high score to zero and exit the program at any time.
- Title screen: A title screen is displayed before the game is started. You can design the title screen freely, but you must include instructions such as "Press any key to start". You may also want to put the how-to-play information within the title screen.
- Characters: There should be 5 moving characters in the game, the Pac-man and four ghosts (in red, pink, cyan and orange).
- Maze and pac-dots: The layout of the maze and all pac-dots should be the same as the screenshot illustrated above. The maze is filled with dots. The small dots are ordinary pac-dots, and the four flashing big pills are power pellets that can temporarily enable the Pacman to eat the ghosts.
- Ghost box: At the center of the maze there is a ghost box which initially constraints three of the four ghosts inside it. Every certain amount of time elapsed, a ghost is unleashed from the box. Also, eaten ghosts go back into the ghost box before they can reappear.
- Messages: On the top of the playfield, there are two labels indicating the current score and the high score. The high score should be kept in a persistent storage (i.e. a file). On the bottom of the playfield, the rest lives is displayed as a corresponding number of Pacmans on the left. Fruit is not necessary in this assignment. Every time a new game is started, a text "READY!" is displayed below the ghost box and a few seconds later it disappears. The characters remain still until the "Ready!" is gone.
Game Rules
Most game rules of the original game can be found in Wikipedia. In this assignment, some of the original rules are eliminated or altered. A list of required rules are as below (bold texts indicate the modified rules for this assignment):
- Basic gameplay: The player controls Pac-man through a maze, eating pac-dots. Four ghosts (known to most gamers as Blinky, Pinky, Inky, and Clyde) roam the maze, trying to catch Pac-Man. If a ghost touches Pac-Man, a life is lost. The initial number of lives is three.
- Power pellets: Near the corners of the maze are four larger, flashing dots known as power pellets that provide Pac-Man with the temporary ability to eat the ghosts. The ghosts turn deep blue, reverse direction, and move more slowly when Pac-Man eats a power pellet. When a ghost is eaten, it turns into a pair of eyes and returns to the ghost home where it is regenerated in its normal color. Blue ghosts flash white before they become deadly again.
- Character behaviors: The ghosts never turn back halfway except when the Pac-Man eats a power pellet. You can simply make their choices random or intelligent (see bonus task 2). Pac-Man moves at a constant speed. Player can use keyboard to control the Pac-Man's moving direction, not speed. If no key is pressed, the Pac-Man continues going forward until it meets a wall and then stops. If two ghosts meet, they keep on moving forward and cross each other.
- Scores: Each pac-dot is worth 10 pts, each power pellets worth 50 pts. With one power pellets, the first ghost eaten is worth 200 pts, and each subsequent worth double (400, 800 and 1600 pts).
- Completing a level: When all pac-dots are eaten, the level is completed and a congratulation screen is displayed within the playfield. If the player's score is higher than the stored high score, the new high score is stored. No signature is needed for the high score. After the player hits any key, the game is reset to the title screen.
- Game over: When all lives have been lost, the game ends and a game over screen is shown in the playfield. If the player's score is higher than the stored high score, the new high score is stored. After the player hits any key, the game is reset to the title screen.
You may add any functionality as you want to improve the specification, but you MUST first implement all the rules listed above as a minimum requirement.
Grading Criteria
Program Functionality (80pt)
- (5pt) Main window: A main window appears after the program is launched. The window contains menu items and a playfield. No other widgets on the window is allowed (any function related to these extra widgets will not be graded).
- (4pt) Menu items: "Reset" menu item restarts the game. "Clear High Score" erases the high score record. "Exit" cause the program to quit. They are functional at any time.
- (5pt) Title screen: When the main window is shown, a title screen is automatically displayed within the playfield. The title screen should contain the name (preferably the logo) of the game, and an instruction telling player how to start and operate the game.
- (15pt) Basic rendering: A maze whose layout (not the graphics) is identical to the screenshot given in this specification is rendered inside the playfield when the game is started with a black background. Five characters, Pac-Man and four ghosts, are drawn inside the maze. Other required maze elements include pac-dots, four larger pac-dots known as power pellets, a ghost box at the center, walls and a tunnel that can teleport characters between left and right edge of the maze. Before a new game is about to start, a "Ready!" message is displayed below the ghost box.
- (4pt) Text: The current player's score and highest scores are displayed at the top of the playfield. In the lower-left corner a number of Pac-Mans is drawn, displaying the number of lives left.
- (6pt) Control: Pac-Man can be controlled by keyboard. It can move in the direction as the player instructs, but the speed is invariant. Pac-Man keeps on moving without changing its direction if there is no operation and stops if it meets a wall.
- (10pt) Basic movement: All characters move along the lanes and cannot go into unreachable areas which are enclosed by the walls. Pac-Man cannot go into the ghost box. Ghosts do not return to the ghost box unlese they are eaten while vulnerable. All characters can pass the teleport tunnel. When a Pac-Man passes a pac-dot (including power pellet), that dot disappears.
- (9pt) Animations: When the characters are moving, they are rendered as animation (changing pictures) instead of still images (e.g.
and ). If the Pac-Man stops moving, its image becomes still. If Pac-Man bumps into a ghost, an animation is played (e.g. ). When Pac-Man eats a power pellet, the vulnerable ghosts turn blue (e.g. ). If a vulnerable ghost is eaten, it becomes a pair of eyes (e.g. ). The vulnerable ghosts will flash before they turn dangerous again (e.g. ). The animations and figures are by no means graded by their aesthetical feeling.
- (10pt) Behavior of enemies: The ghosts keep moving in the same direction until they reach a cross where they can make a random choice, except when Pac-man eats a power pellet and they all turn back immediately and slow down. If a vulnerable ghost is eaten, it turns into a pair of eyes and seek its way back to the ghost box, but not necessarily the shortest way. The effect of power pellet lasts at least 5 seconds but no more than 10 seconds.
- (6pt) Game status: Game score, high score and lives left are updated and rendered correctly. The high score is kept in a persistent way so that it won't be lost as the program exits. Game status is initialized whenever a new game is started.
- (6pt) Gameovers: When all pac-dots are eaten, a congratulation screen is displayed. If all lives are lost, a game over screen is displayed. In either case, the player can dismiss the screen by hitting a key and the game goes back to the title screen.
Program Readability (20pt)
Code Standards (6pt)
- (3pt) The code is formatted uniformly (use the function in your IDE). Classes, objects and members are named nominatively. This is a C++ coding standard for your reference.
- (3pt) There should be explanatory comments to help readers understand your code. Give a brief description about the class at the beginning of each class file.
Documentation (14pt)
- (4pt) Explain what the classes and objects are involved in this program and their functions and give necessary UML diagrams. You should particularly focus on inheritance and polymorphism (if there is any), explaining how and why you use them.
- (2pt) Explain how you migrated the application from the Java example to C++/QT and what is rewritten or appended.
- (3pt) Explain with sufficient details the data structure you used for storing pac-dots, maze, etc.
- (2pt) Explain the algorithms related to path finding (e.g. path finding for an eaten ghost to return to the ghost box).
- (3pt) Explain your implementation for animation, double buffering, and other rendering issues. Specify the classes and objects you used in relation to these issues.
In addition to the above checkpoints, please list everything you have implemented in your documentation. If something is implemented in the source code but not mentioned in your documentation, it is not graded and you will not receive any point.
Your documentations can be in one of two formats (1) PDF or (2) README. A Word file is not accepted because there are too many versions of Word programs and the formatting can be different. README is an ASCII file and the name must be README. This is a widely accepted convention by millions of people. The file name is not readme, readme.txt, Readme, or anything else. It must be README. If you writes README file and what to include UML diagrams, you can submit the UML diagrams as separated PDF files. Any other formats, such as pictures, Visio files, NetBeans diagrams etc., are not accepted. You can use "Print to File" or PDFCreator to export your diagrams as PDF files.
If you submit a PDF documentation, please embed the UML diagrams into the documentation.
Avoid plagiarism: Whenever you cite text, pictures, ideas, etc., be sure you include the source in your reference. This includes information from the Internet.
Bonus (20pt)
- (3pt) Demo mode (a.k.a attract mode): When a title screen is idle for a long time, the game enters demo mode, in which the Pac-Man is controlled by computer. No AI is needed for the computer player. Whenever a key is pressed in demo mode, the game returns to the title screen.
- (5pt) Intelligence of the ghosts: Implement AI for the red and the pink ghosts. The red ghost (Blinky, chaser) constantly tries to find a shortest way approaching the back of the Pac-man; the pink ghost (Pinky, ambusher) tries to find a shortest way to the front of Pac-man. They are still disallowed to change their direction halfway until they reach a cross. There is no need to prevent the red ghost from being an ambusher where necessary, or vise versa. Their strategies are illustrated below. There is no spefication for the orange and cyan ones. Documentation for explanation of the implemented algorithm is crucial to this bonus task.
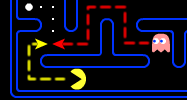
(Note: This moving pattern has nothing to do with the personalities of the ghost in the original official game.)
- (12pt) Dynamic maze generation: There should be a menu item activating the function of dynamic maze generation. A dynamically generated maze should satisfy the following conditions:
- Five characters as usual
- There is a ghost box at the center.
- All passages should be at unit width, i.e. the Pac-Man and the ghosts can only move along the passages.
- There must be a tunnel, regardless of whether horizontal or vertical, which can teleport the characters from one edge to the opposite.
- Most of the reachable area should be filled with pac-dots, at least one of them being a power pellet.
- Any wall should enclose a certain unreachable area. This means that there must not be broken walls in the maze.
- It is possible to win, i.e. to eat all pac-dots.
You must describe the algorithm of maze generation in your documentation, otherwise this bonus task will not be graded. If the dynamic generation procedure makes use of some pre-designed and pre-stored maze patterns (which means the maze is not purely dynamic), the bonus will be discounted.
Supplementary Material
Here is a sprite set from the original Pac-Man arcade game by Namco. This is only for your reference and you do not have to use it. Since this is a copyrighted material, please do not use it for purposes other than this assignment. You need to divide, resize and make transparent the following image by yourself. You can use external image editors, such as GIMP, or keep it as a whole image and "divide" it within your code.
|