ECE 462 Assignment 3
Tetrix game using Java (outcome 1)
In this assignment, you can use the Tetrix game in Qt as a reference. Your program must use Java. If your assignment 1 does not pass outcome 1, this is the second and final chance.

Tetrix is an interactive game. You can find the rules at Wikipedia. This assignment gives you an implementation written in C++ and Qt. You need to convert it to Java and expand it to a 2-player game. You can obtain the source code from Qt or use the following command:
cp -r /home/shay/a/sfwtools/public/qt4.3.0/examples/widgets/tetrix/ .
@msee190pcx (x = 1 ~ 20). Please remember to use Qt 4.x. Qt 4.x is available @msee190pcx.ecn.purdue.edu, @algoly.ecn (y = 1 ~ 20), or @qstructz.ecn (z = 01 ~ 19), or the Linux computers in ENAD. Qt 4.x does not run on Sun computers. You need to ssh into one of the Linux computers.
To compile the program, type
qmake -project
qmake
make
To execute the program, type
./tetrix
Two-Player Tetrix
This specification is given "as it is.". If anything is incomplete, inconsistent, or incorrect, please explain your interpretation or improvement of the specification in README. One of the purposes of this assignment is to provide an opportunity to learn how to handle an imperfect specification. You can discuss how to improve the specification by posting in Blackboard discussion.
This is an extension of the one-player game. Two play fields are horizontally displayed.
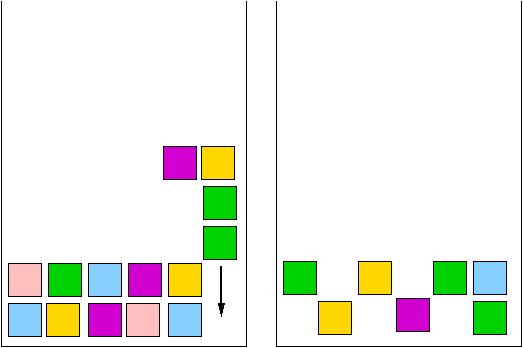
When one line is filled, the line except the last square or squares to fill that line, is moved to the other player.
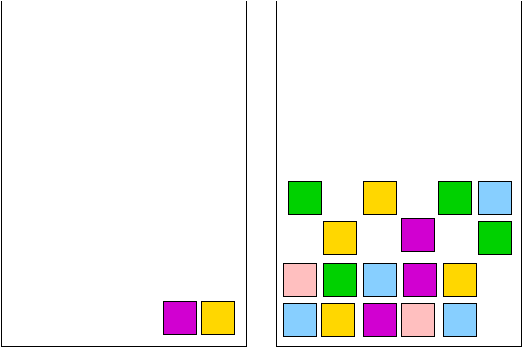
Another example is shown below.The bottom two lines are move to the right player's field.
becomes 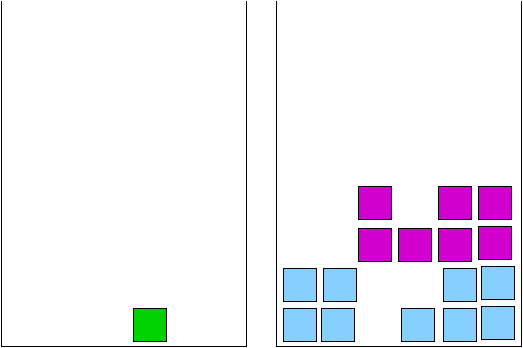
Game Components
The game includes the following components:
- Play fields: There are two play fields side-by-side. The width of each field should be 10 squares. The height is 24 squares.
- Tetrix piece: There are seven types of Tetrix pieces. Each type has a different color. Each piece has four squares. Tetrix pieces fall from the top and move to the bottom. The pieces in the two play fields can be different. (A simple solution is to use two random numbers to determine which pieces to use.)
- Menu: There is a menubar and a menu. The menu contains at least five menu items: Demo, One Player, Two Players, Save, and Open.
- Demo mode: both fields are controlled by computers.
- One Player: the left field is controlled by a person and the right field is controlled by the computer. The person controls the movements and rotations using the keyboard.
- Two Players: the two fields aer controlled by two people. They use different keys to control the movements and rotations of Tetrix pieces.
- Save in the Play mode: FileChooser appears and asks the user to provide a file name. The current status of the game is saved in the file. Your program should not respond if the user clicks Save in the Demo mode.
- When a user clicks Open and the game is in the Demo mode, the game automatically enters the Play mode, shows Q and reads the game status from the file. The game resumes from the previously saved status.
- If the game is in the Play mode and the user clicks Open, the program creates a FileChooser dialog to ask whether the current game status should be saved. The user has three options: Yes, No, and Cancel. If the user selects Yes, the program first asks for a file name to save the current status and then asks for another file name to retrieve the previously saved status. If the user selects No, the program discards the current status and asks for another file name to retrieve the previously saved status. If the user selects Cancel, the program returns to the condition before Open was selected.
The game is frozen (i.e. the pieces stop falling) when the menu is clicked. You are encouraged to add more menu items as part of the program development.
- Status labels: There are four status labels, two for each player, to show
- Scores: every time a horizontal line is eliminated, the player's score increases by one.
- The shape of the next piece.
Grading Criteria
Program Functionality (80pt)
- (6pt) A main window appears after the program is launched. The window contains (at least) four labels, two panels and a menubar containing five menu items (Demo, One Player, Two Players, Save, and Open). You can rearrange the menu items into different levels. All labels should contain descriptive text ("Next", "Score", etc.).
- (6pt) Seven types of Tetrix pieces are designed in different colors and appear in the game.
- (8pt) Human player can use keyboard to operate the falling pieces. You must show the operating instructions somewhere in your program (e.g. in a splash window, or at the bottom of the game window). At least the following actions are implemented: moving left/right, rotating, accelerating down (must be "soft drop". See the definition in Wikipedia).
- (6pt) Rotation is processed correctly. Player cannot rotate a piece if it collides with an existing square or the wall. Rotation will never cause a piece to go up (this would considered as a bug).
- (6pt) When a piece falls on the stack, it is fixed and the next piece appears at the top. The next piece label is then updated to a new piece. Pieces are not allowed to move across the boundary of the playground.
- (8pt) When a horizontal line is full, it is eliminated and the score is updated correctly. The eliminated squares, except the most recently added, are moved to the opponent's field at the bottom according to the given rule.
- (6pt) When elimination occurs, squares above the eliminated lines fall down due to gravity. The effect of gravity is illustrated as below:

- (5pt) If the upcoming piece collides with existing pieces in the playground, the game is over. A message indicates the winner.
- (8pt) When Save menu is clicked, a Save dialog appears. After the user chooses a file name, the game status is saved; When Open menu is clicked, an Open dialog appears. After the user specifies a file, the saved status is restored. The correctness of Save and Open functions is graded together. The functions are tested in the way that a status is first saved and then opened. You can receive the points for Open only if you have implemented Save correctly.
- (6pt) In Demo mode, both side are controlled by the computer. In One Player mode, the left side is controlled by human and the right side by computer. In Two Players mode, both are controlled by human (both with keyboard, but different keys). In Demo mode, Save is disabled. In other modes, Save and Load are enabled.
- (5pt) Games are initialized and started automatically when 1) user switches among Demo, One Player and Two Players modes 2) user dismisses a Game Over message.
- (10pt) The computer player can move and rotate 90% pieces correctly to eliminate existing lines.
Program Readability (20pt)
Code Standards (5pt)
- (2pt) The code is formatted uniformly (use the function in your IDE). Classes, objects and members are named under Java programming conventions.
- (3pt) There should be explanatory comments to help reader understand your code. Give a brief description about the class at the beginning of each class file.
Documentation (15pt)
- (4pt) Explain what the classes and objects are involved in this program and their functions and give necessary UML diagrams. You should particularly focus on inheritance and polymorphism (if there is any), explaining how and why you use them.
- (2pt) Describe the interactions among the classes and the objects in UML.
- (3pt) Explain how you migrated the application from Qt to Java -- what remains unchanged and what is rewritten or appended.
- (4pt) Analyze the game algorithms such as rotation, collision, eliminiating lines, etc. with sufficient details.
- (2pt) Explain the structure of the status files for Save and Open functionality.
In addition to the above checkpoints, please list everything you have implemented in your documentation. If something is implemented in the source code but not mentioned in your documentation, it is not graded and you will not receive any point.
Your documentations can be in one of two formats (1) PDF or (2) README. A Word file is not accepted because there are too many versions of Word programs and the formatting can be different. README is an ASCII file and the name must be README. This is a widely accepted convention by millions of people. The file name is not readme, readme.txt, Readme, or anything else. It must be README.
Avoid plagiarism: Whenever you cite text, pictures, ideas, etc., be sure you include the source in your reference. This includes information from the Internet.
Bonus Points (15pt)
- (3pt) Dual launch: make your program launchable as both an application and an applet (such that a web browser can view it). To show you have implemented this function, place the same Java class file and a web page, which refers to this class as an applet, onto your homepage space (if you have access to ECN, you can utilize your personal folder), then provide the URL to this web page in README. The functionality of the applet should be exactly the same as that of the application. This online tutorial is for your reference.
- (3pt) Implement the two gravity modes as described in Wikipedia (pay attention to the illustrations there). User can switch between the two modes through a checkbox menu item.
- (3pt) Implement the function that allows the player to configure their operating keys. A menu item named "Define Keys..." in the main window activates a dialog box in which the player can define keys (left/right/accelerate/rotate).
- (6pt) Record Game: Two menu items, "Record" and "Playback", activate the record/playback functionality. When Record is clicked, a Save dialog appears for user to specify a new file for recording. After the user chooses a file name, the current play mode is restarted and any subsequent moves, regardless of whether by computer or by human, are recorded to that file until the game is over or interrupted (e.g. by mode switch or program termination). The playback function should allow user to reproduce the exact scenes recorded previously from a file. You must explain your implementation, as well as the structure of a recording file, in your documentation with sufficient details, otherwise the implementation will not be graded.
Frequently Asked Questions
Q: Is rotation determined by path or destination? Can a piece rotate if the final desination allows it but its square collides with another piece during rotation?
A: Rotation is determined by path. If collision occurs, the piece cannot rotation, even if the destination can accommodate the piece. In the following example, the green piece cannot rotate.
This rotation is not allowed because collision occurs during rotation, even though the destination can accommodate the piece.
Q: When are the eliminated squares moved to another field? Immediately? What if it will cause the stack to overlap with a falling piece?
A: Eliminated squares are moved to another field immediately. However, if this will cause the raised stack to overlap a falling piece, the moving action is delayed until the next piece is about to appear.
|