ECE 462 Assignment 1
Two-player Breakout game using Java (outcome 1)
Please finish the first two lab exercises before starting this programming assignment.
Breakout Game
In this assignment, you will develop a Java Breakout Game. This game has many variations and some are available on line without charge, for example
 
You will learn how to create a computer game in Java, including how to
- create a graphical user interface.
- handle user inputs.
- perform collision detection.
- save and retrieve game status using files.
Since this is the first Java program for many of you, you you must write the program "from scratch." Do not download any open-source program and modify it for this assignment. This specification is given "as it is.". If anything is incomplete, inconsistent, or incorrect, please explain your interpretation or improvement of the specification in README. One of the purposes of this assignment is to provide an opportunity to learn how to handle an imperfect specification. You can discuss how to improve the specification by posting in Blackboard discussion.
Game Components
This is a variation from the traditional one-player game. The assignment requests that you write a game that allows a person to play against a computer. The following figure is for your reference. Your program may put the playground above or below the game status.
The game includes the following components.
- Playground: It contains two paddles, the ball, and the bricks.The size of the playground should be 400 (width) x 300 (height) pixels. On a computer screen, the origin is at the upper left corner.
- Paddles: There are two paddles in the game. Both move vertically. The left one is controled by the computer. The right one is controled by the human player; its movement follows the mouse's movement.
- Bricks: They are at the middle of the playground and arranged into grids. You can decide whether the bricks are arranged in any shape other than a rectangle. There should be at least 1 pixel gap between bricks. The actual sizes of bricks and gaps are left for your decision. You can have bricks of different sizes as well but this will make collision detection more complex. There should be at least 25 bricks when the game starts.
- Ball: It bounces after hitting one of the paddles, a boundary of the playground, or a brick. The bounce (also called reflection) follows the law of reflection: the angle of incidence equals the angle of reflection. If the ball falls below (above) the x coordinate of the left (right) paddle, the ball is lost and the number of ball decreases by one. Please be aware that it is possible for the ball to hits two bricks simultaneously, as below. The size of ball should be at least 10-pixel wide (radius = 5).
- Menu: There is a menubar and a menu. The menu contains at least four menu items: Demo, Play, Save, and Open.
- Demo mode: the right paddle tracks the movement of the ball and reflects the ball back to the playground. Please notice that the paddle must not strictly follow the ball and it must be possible to lose the ball in the demo mode.
- Play mode: a human player has to move the right paddle by moving the mouse up or down. If the paddle at the top of the playground, moving the mouse up has no effect to the paddle's position. Similarly, if the paddle is at the bottom of the playground, moving the mouse down has no effect to the paddle's position.
- Save in the Play mode: a FileChooser dialog appears and asks the user to provide a file name. The current status of the game is saved in the file. Your program should not respond if the user clicks Save in the Demo mode. More details about Java's FileChooser can be found here.
- When a user clicks Open and the game is in the Demo mode, the game automatically enters the Play mode, shows a FileChooser dialog and reads the game status from the file. The game resumes from the previously saved status.
- If the game is in the Play mode and the user clicks Open, the program creates a dialog to ask whether the current game status should be saved. The user has three options: Yes, No, and Cancel. If the user selects Yes, the program first asks for a file name to save the current status and then asks for another file name to retrieve the previously saved status. If the user selects No, the program discards the current status and asks for another file name to retrieve the previously saved status. If the user selects Cancel, the program returns to the condition before Open was selected.
The game is frozen (i.e. the ball does not move) when the menu is clicked. You are encouraged to add more menu items as part of the program development, for example, one menu item to test the reflection of the ball when it hits the paddle.
- Status labels: There are four status labels to show
- Score: every time the ball bounces against a wal, the score increases by one for the paddel that had the latest collision with the ball. If the ball bounces against a brick, the score increases by two for the paddel that had the latest collision with the ball. If the ball hits the left wall, the ball is lost and the computer loses 10 points. If the ball hits the right wall, the ball is lost and the player loses 10 points.
- Ball's position, as (x, y), in the unit of pixels.
- Number of balls left: Each game has six balls. When no ball is left, the game is over.
- Paddle's position, as y, in the unit of pixels. Please select a representative point, such as the top of the paddle, for the position.
- You can display other game status as well, for example, the current velocity of the ball, the current mode (Demo or Play), and the location of the last collision with the paddle.
- Speed control: a horizontal slider is used to control the speed of the ball. The ball's speed is higher when the slider's location is at the right end. To make the game "playable", the ball must move within reasonably speeds. When the slider is at the left end (the lowest speed), the ball must move slower, without stopping. At the highest speed, the ball takes approximately 2 seconds to cross the playground's width.
- Suppose all surface are rigid, i.e. their shapes do not change during collisions.
Programming Environment in MSEE 190
Several programming tools are installed and available for you to use. For details, please read /home/shay/a/sfwtools/public/README. To use these tools, you need to set the paths correctly. If you use tcsh, you can simply add the following line
source /home/shay/a/sfwtools/public/settings
in your .cshrc. You need to use an ee462xxx account to execute these programs. This account is given to you through Blackboard. Do not use your personal Purdue account as some tools are available to ee462xxx accounts only.
Program Functionality (80pt)
- (10pt) A main window appears after the program is launched. The window contains (at least) four labels, a panel (400x300, for playground), a horizontal slider and a menubar containing four menu items (Demo, Play, Save, and Open). Each label should contain a descriptive text.
- (8pt) When a new game is started, the playground should contain two arc paddles (with margins of at least 5 pixels to the borders), a ball (at least 10-pixels wide) and at least 25 bricks with gaps between them.
- (7pt) The ball bounces correctly when hitting the paddle and top and bottom borders of the playground.
- (15pt) The ball bounces correctly when hitting the brick(s). The hit brick is removed. You must consider the situation of the ball hitting a corner of a brick (a bounding box of any shape may cause the collision to be detected prior to the actual occurrence, thus you cannot use bounding-box approximation for collision detection between the ball and a brick). If a ball goes behind the paddle, the ball is lost, and scores and number of balls left are correspondingly updated. A new ball is then appears in the playground, going towards the opponent, and before it reachs a paddle, it cannot hit any brick (to avoid the situation that no one can claim the score of the hit bricks).
- (3pt) When user drags the slider, the velocity of the ball should change accordingly, within the specific range.
- (5pt) Human player can control the right paddle by mouse movement in Play Mode.
- (4pt) Labels track the ball's position, the paddles' positions, the scores and the number of balls left. Scores are updated correctly.
- (4pt) Game is over when all bricks are removed or no ball is left. A message indicates the winner.
- (6pt) When Save menu is clicked, a Save dialog appears. After the user chooses a file name, the game status is saved.
- (6pt) When Open menu is clicked, an Open dialog appears. After the user specifies a file, the saved status is restored. The correctness of Save and Open functions is graded together. The functions are tested in the way that a status is first saved and then opened. You can receive the points for Open only if you have implemented Save correctly.
- (7pt) A paddle controlled by the computer can hit the ball at a successful rate greater than 80% but it must be possible to lose the ball. The computer-controlled paddle must move at a reasonable speed (at most twice as fast as the ball).
- (5pt) The game is frozen when a menu is selected and resumed when the menu is deactivated. The game is initialized when mode is changed, switching between Play and Demo modes works properly.
Program Readability
Code Standards (8pt)
- (3pt) The code is formatted uniformly (use the function in your IDE). Classes, objects and members are named under Java programming conventions.
- (5pt) There should be explanatory comments to help reader understand your code. Give a brief description about the class at the beginning of each class file. Comment wisely: You should place proper comments at codes that are not easy to understand. Do not comment self-explanatory codes.
Documentation (12pt)
- (4pt) Explain what the classes and objects are created for this program and the reasons. You should particularly focus on inheritance and polymorphism (if there is any), explaining how and why you use them.
- (2pt) Describe the interactions among the classes and the objects.
- (4pt) Analyze the collision detection algorithm and explain the implementation of bouncing and reflection.
- (2pt) Explain the structure of the status files for Save and Open functionality.
Please list everything you have implemented in your documentation. If something is implemented in the source code but not mentioned in your documentation, it is not graded and you will not receive any point.
Your documentations can be in one of two formats (1) PDF or (2) README. A Word file is not accepted because there are too many versions of Word programs and the formatting can be different. README is an ASCII file and the name must be README. This is a widely accepted convention by millions of people. The file name is not readme, readme.txt, Readme, or anything else. It must be README.
Avoid plagiarism: Whenever you cite text, pictures, ideas, etc., be sure you include the source in your reference. This includes information from the Internet.
Bonus Points (maximum 20pt)
Bonus points are obtained by implementing any of the following functions.
- (3pt) Sound is played (1) when the ball hits a paddle or a brick (2) when a player loses a ball (3) when game is over (4) when a new game starts. Sound can be turned on or off through user interface.
- (5pt) There are several kinds of bricks. Different kinds of bricks have different sizes. If you use images, you will receive more points.
- (12pt) Create a Super mode in which the computer player has a perfect strategy to keep the ball at its side until all bricks are removed (see supplementary materials). You must elaborate, in your documentation, your mathematical and algorithmic analysis to implement this strategy.
Supplementary Materials
Computer Coordinates: If you have not written any program using graphics, you may not know that in a program, the origin is at the left upper corner, as shown below.
Simultaneous Collision: It is possible that the ball hits two bricks simultaneously, as shown below.
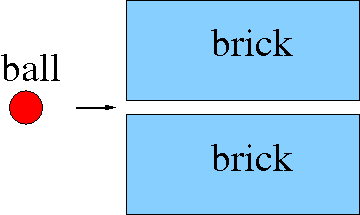
Law of Reflection: Suppose Vi is the ball’s original direction and N is the normal vector. The ball’s new direction is Vo. The angle between N and Vi is called the angle of incidence alpha ; the angle between N and Vo is called the angle of reflection beta. The law says that alpha = beta.
Normal Vector of Paddle: It is easy to calculate the normal vector of the paddel. It is the direction from the arc’s center C to the location of collision.
How to calculate the reflected direction Vo?
The sum of Vo and (-Vi) is a vector in the same direction as N. Therefore, we can write
Vo - Vi = sN,
here s is twice Vi's projection on N. In other words, s is twice the inner product between (-Vi) and N, namely 2 (-Vi) N.
Vo = Vi - 2 (Vi N) N
Let Vi = (xi, yi), N = (xn, yn), and Vo = (xo, yo).
xo = xi - 2 (xi xn + yi yn) xn
yo = yi - 2 (xi xn + yi yn) yn.
Java fillArc
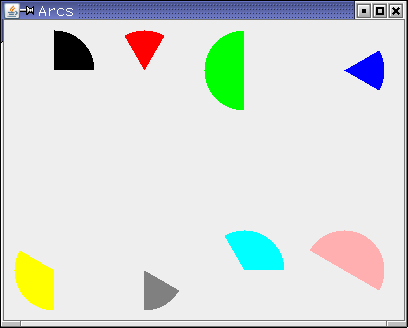
gfx.setColor(Color.BLACK);
gfx.fillArc(10, 10, 80, 80, 0, 90);
gfx.setColor(Color.RED);
gfx.fillArc(100, 10, 80, 80, 60, 60);
gfx.setColor(Color.GREEN);
gfx.fillArc(200, 10, 80, 80, 90, 180);
gfx.setColor(Color.BLUE);
gfx.fillArc(300, 10, 80, 80, 330, 60);
gfx.setColor(Color.YELLOW);
gfx.fillArc(10, 210, 80, 80, 150, 120);
gfx.setColor(Color.GRAY);
gfx.fillArc(100, 210, 80, 80, 270, 60);
gfx.setColor(Color.CYAN);
gfx.fillArc(200, 210, 80, 80, 0, 120);
gfx.setColor(Color.PINK);
gfx.fillArc(300, 210, 80, 80, 330, 180);
You can see a figure explaining the definition of the parametes here. You can find the document.
Java Arc2D
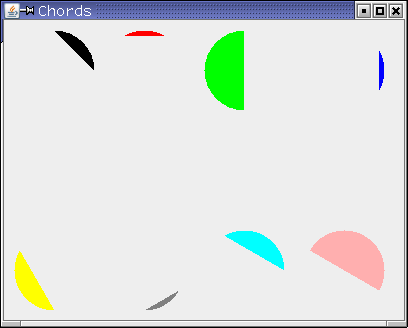
public void paintComponent(Graphics gfx) {
Graphics2D g2d = (Graphics2D) gfx;
gfx.setColor(Color.BLACK);
Arc2D ch1 = new Arc2D.Double();
ch1.setArc(10, 10, 80, 80, 0, 90, Arc2D.CHORD);
g2d.fill(ch1);
gfx.setColor(Color.RED);
Arc2D ch2 = new Arc2D.Double();
ch2.setArc(100, 10, 80, 80, 60, 60, Arc2D.CHORD);
g2d.fill(ch2);
gfx.setColor(Color.GREEN);
Arc2D ch3 = new Arc2D.Double();
ch3.setArc(200, 10, 80, 80, 90, 180, Arc2D.CHORD);
g2d.fill(ch3);
gfx.setColor(Color.BLUE);
Arc2D ch4 = new Arc2D.Double();
ch4.setArc(300, 10, 80, 80, 330, 60, Arc2D.CHORD);
g2d.fill(ch4);
gfx.setColor(Color.YELLOW);
Arc2D ch5 = new Arc2D.Double();
ch5.setArc(10, 210, 80, 80, 150, 120, Arc2D.CHORD);
g2d.fill(ch5);
gfx.setColor(Color.GRAY);
Arc2D ch6 = new Arc2D.Double();
ch6.setArc(100, 210, 80, 80, 270, 60, Arc2D.CHORD);
g2d.fill(ch6);
gfx.setColor(Color.CYAN);
Arc2D ch7 = new Arc2D.Double();
ch7.setArc(200, 210, 80, 80, 0, 120, Arc2D.CHORD);
g2d.fill(ch7);
gfx.setColor(Color.PINK);
Arc2D ch8 = new Arc2D.Double();
ch8.setArc(300, 210, 80, 80, 330, 180, Arc2D.CHORD);
g2d.fill(ch8);
}
The document of Java Arc2D is here.
Super Mode Game Strategy: It is possible to create an unbeatable player that controls the ball's direction so that the opponent never has a chance to hit the ball. The trick is using the curve surface of the paddle to control the ball. For example, the paddle makes the ball move horizontally to hit a brick. If the paddle controls the ball's direction and aims directly the left side of the brick, the ball bounces back to the paddle. After the ball hits the brick, the paddle aims at another brick and makes the ball hit the left side again.
Maintain Readable Source Codes: It is essential to keep the readability of the source codes in a large-scale software project. Documentations and programming comments are two extremely important supports for program readability. Therefore, we have some relevant requirements in your assignment. You may want to check the following websites to have a brief idea of how to maintain qualified documentations, comments and code styles.
|